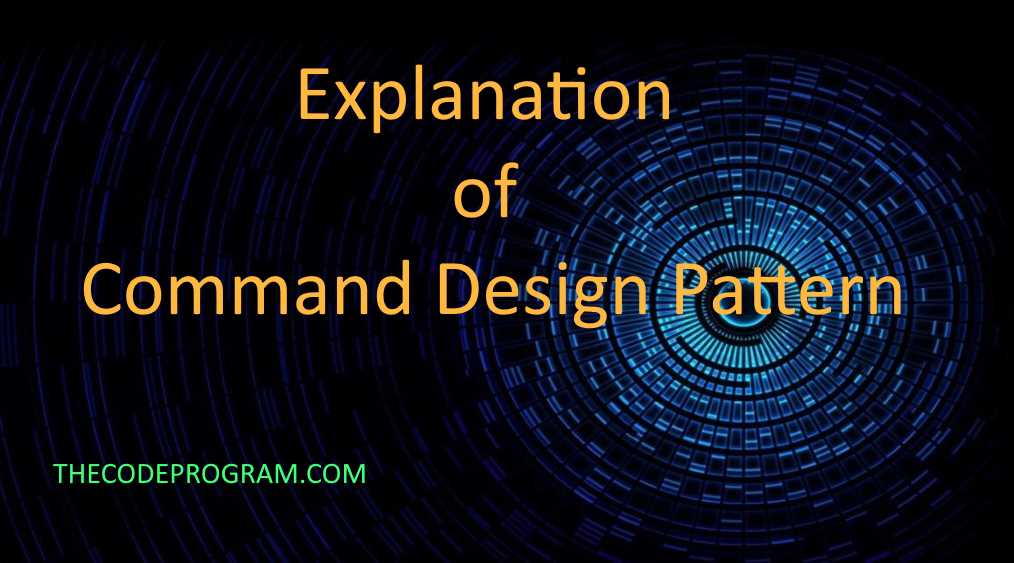
Explanation of Command Design Pattern
Hello everyone, in this article we are going to talk about Command Design Pattern which is part of Behavioral Design Patterns. First ı will give a description and later I will give an example about Command Design Pattern.Now Let's Begin.
Firstly What is Command Design Pattern:
A request will be wrapped under an object and after be sent to an invoker. And after will be executed this request. Sometimes your program can be frezed and at this time another thread may fire another code. During this frezing time your program can miss any other datas or commands. To prevent this we can keep all of these commands inside an invoker and our invoker will be fire them one by one.
For another example, when you type something on your text editor, your computer or your text editor program may be frezed. If the provider of the editor build the program with the command design pattern and that program keep the typed characters and insert them one by one, your program will never lose the typed characters. It will keep the inserting the data when come back.
Command design pattern is the way to do this.
Below example you can see my example:
First I will create a command Selector and two more command classes. We will select the commands through the selector class.
Below code block you can see the my selector and command classes. I will create aircraftSelector class and two aircraft brands named Boeing and Airbus.
IAircraftSelector.cs
namespace CommandPattern_Example
{
interface IAircraftSelector
{
string AircraftName { get; }
void SelectAircraft();
}
}
Airbus.cs
using System;
namespace CommandPattern_Example
{
class Airbus : IAircraftSelector
{
public string AircraftName
{
get
{
return "Airbus";
}
}
public void SelectAircraft()
{
Console.WriteLine("Selected Aircraft is Airbus");
}
}
}
Boeing.cs
using System;
namespace CommandPattern_Example
{
class Boeing : IAircraftSelector
{
public string AircraftName
{
get
{
return "Boeing";
}
}
public void SelectAircraft()
{
Console.WriteLine("Selected Aircraft is Boeing");
}
}
}
Now we have to build our Command Selector Class. In this case I created the AircraftSelector interface and here we create this related CommandInvoker class and we will make our invokes from mainprogram through this AircraftSelector by CommandInvoker class.
CommandInvoker.cs
namespace CommandPattern_Example
{
class CommandInvoker
{
IAircraftSelector selector;
public IAircraftSelector getAircraft(string _aircraft)
{
if (_aircraft.Equals("Airbus"))
selector = new Airbus();
else if (_aircraft.Equals("Boeing"))
selector = new Boeing();
return selector != null ? selector : null;
}
}
}
Program.cs
using System;
namespace CommandPattern_Example
{
class Program
{
static void Main(string[] args)
{
//Create the commandInvoker and AircraftSelector interface objects
CommandInvoker cmdInvoker = new CommandInvoker();
IAircraftSelector selector;
//Here we selected the Airbus aircraft
selector = cmdInvoker.getAircraft("Airbus");
selector.SelectAircraft();
//Here we selected the Boeing aircraft
selector = cmdInvoker.getAircraft("Boeing");
selector.SelectAircraft();
//Wait for any key to press
Console.ReadLine();
}
}
}
That is all in this article.
Have a good Commanding to your classes.
Burak Hamdi TUFAN
Comments