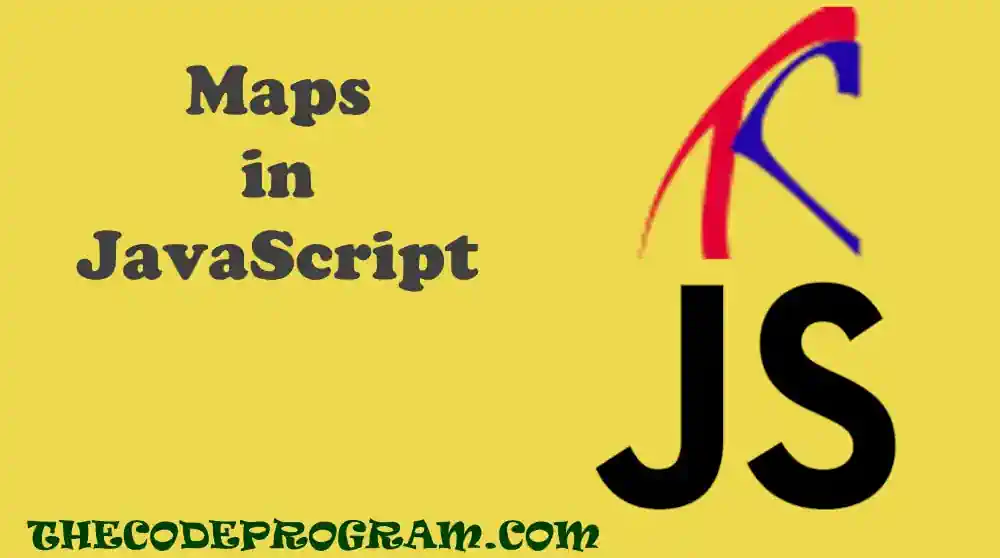
Explanation of Map in JavaScript
Hello Everyone, in this article we are going to talk about Map structure in JavaScript. We will make explain and make some examples for better understanding of Maps in JavaScript.Let's get started
What is Map?
Map is a powerful data structure to store values as a key-value pairs and allows us to access these values with unique keys. Maps are introduced for JavaScript with ES6 stardarts.
We can create and initialize a map with new keyword. Below you can see how to create a Map structure in Javascript
const theMap = new Map();
We can also initialize a Map with some initial values. Below you can see how to initialzie a Map with initial values.
const theMap = new Map([
['key1', 'val1'],
['key2', 'val2'],
]);
We can add key-value pair with set method and we can access the value by key with the get method. Below you can see examples for adding and reading data.
const theMap = new Map();
theMap.set('name', 'Burak');
theMap.set('lang', 'JavaScript');
theMap.set('experience', 17);
console.log(theMap.get('name'));
console.log(theMap.get('lang'));
console.log(theMap.get('experience'));
Below picture you can see the execution of above code block.
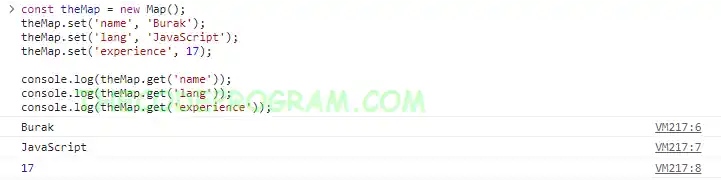
We can access the key-value pairs together as an entry. Also we can access the array of the all keys and array of only values. For these operations we will need entries(), keys() and values() functions of the Map class. Now lets make some examples.
const theMap = new Map();
theMap.set('name', 'Burak');
theMap.set('lang', 'JavaScript');
theMap.set('experience', 17);
const entryPairs = theMap.entries(); //Array of [key, value]
const arrayOfKeys = theMap.keys();
const arrayOfValues = theMap.values();
console.log('List of key-value pairs');
for (const [key, value] of entryPairs ) {
console.log(`${key} -> ${value}`);
}
console.log('List of keys');
for (const key of arrayOfKeys ) {
console.log(`${key}`);
}
console.log('List of Values');
for (const value of arrayOfValues ) {
console.log(`${value}`);
}
Execution output will be like below
List of key-value pairs
name -> Burak
lang -> JavaScript
experience -> 17
List of keys
name
lang
experience
List of Values
Burak
JavaScript
17
That is all for this article about using Map in Java Script.
Burak Hamdi TUFAN
Comments