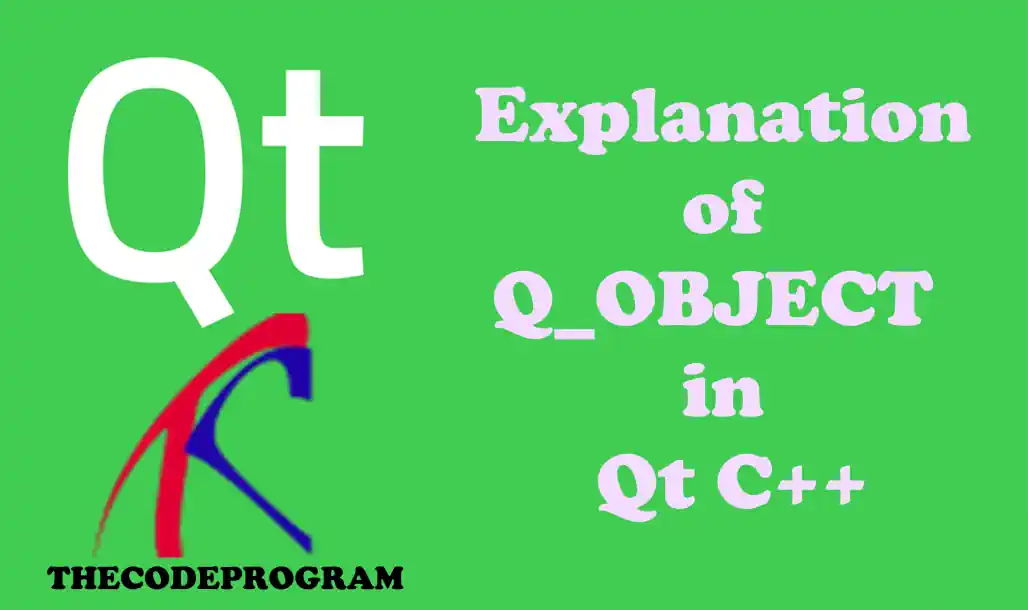
Explanation of Q_OBJECT macro in Qt C++
Hello everyone, in this article we are going to talk about Q_OBJECT which is one of most important macros of Qt framework. We will see the usages and make some examples for better understanding.Let's get started.
What is Q_OBJECT ?
Q_OBJECT is one of the core macros of Qt Framework. Q_OBJECT allows us to use some important features of Qt meta object system, signal-slot mechanism is one of them. To enable these features, we add Q_OBJECT definition to the begining of the class.
#include <QObject>
class MyObject : public QObject
{
Q_OBJECT
// public and private class definitions...
public slots:
void calculate();
signals:
void errorOccured();
};
As you can see above, We added Q_OBJECT at the begining of the class definition. To be able to add this macro, this class should inherit the QObject class. Also as you can see we are able to define the signals and slots in this class. With Q_OBJECT macro this class is a meta object class now and at the compiling time Meta-Object system of Qt will rewrite this class.
With Q_OBJECT macro also Property System will be enabled.
With Property system we are able to enable some of the events after of a class members' read, write and more events...
#include <QObject>
class MyObject : public QObject
{
Q_OBJECT
Q_PROPERTY(QString value READ getValue WRITE setValue NOTIFY valueChanged)
public:
explicit MyObject(QObject *parent = nullptr);
QString getValue() const;
void setValue(const QString &name);
signals:
void valueChanged();
private:
QString m_value;
};
As you can see above, we defined m_value variable and we bound the getter, setter and signal to this value on the events read, write and changed. Property system is being enable by Q_OBJECT macro.
That is all for this article
Burak Hamdi TUFAN
Comments