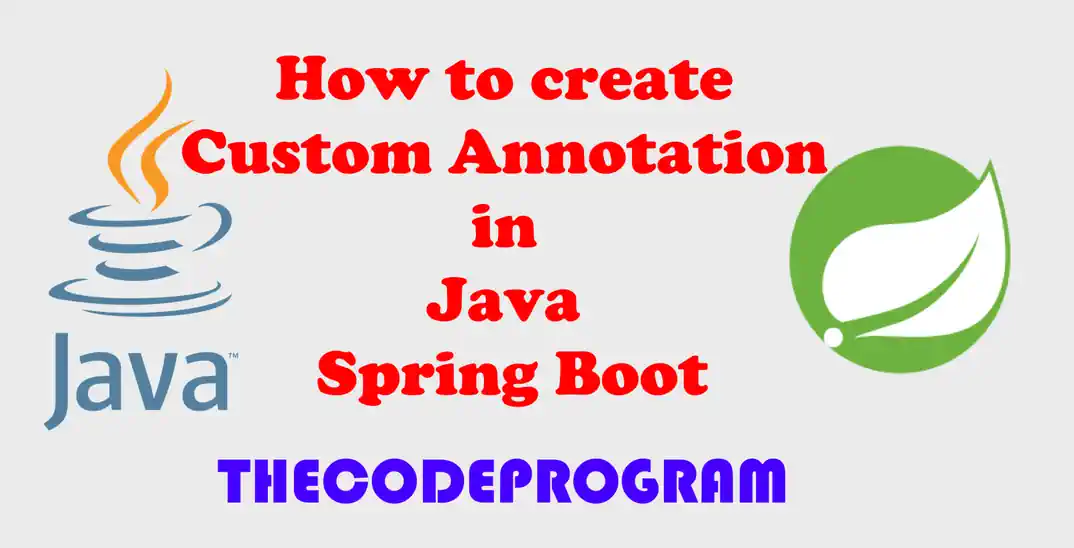
How to create Custom Annotation in Java Spring Boot
Hello everyone, In this article we are going to talk about Annotations in Java and we will we are going to create our own annotation to improve code in Spring boot.Let's get started
What is the annotation in Java?
Annotations provide metadatas for the code blocks. With annotation some additional informations will be passed to compiler and these informations are being used for compiling for sometimes they are working also at runtime and we can perform some operations.
If we can give an example for usages, we can define an annotation to limit the access to a method according to user authentication level. We can perform some of the pre-checks by the help of annotations. As we know springboot is using annotations mainly to reduce complexity and improve the code quailty and maintainability.
We put annotations before or above line of decleration and annotations starts with @. Below you can see an the syntax:
@Annotation
public void methodName(...){
//......
}
Now lets create our own annotation in Java SpringBoot
Firstly we need to create an interface for for the annotation BUT this is not a regulare interface so we will need to define it with @interface keyword. With this decleration also we will need to specify our annotation configurations like target element type or where it will be considered.
Below you can see the decleration of an annotation interface.
package com.thecodeprogram.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface CheckLevel {
int level() default 0;
}
In above example as you can see we have created a an annotation which named as CheckLevel and it is taking parameter as level. Also we defined default value for level is 0. Also we defined target as element is methods and it will be there during the program Runtime also.
So now we need to create the logic for this annotation to define what it will do.
package com.thecodeprogram.annotation;
import java.lang.reflect.Method;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.reflect.MethodSignature;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class CheckLevelLogic {
ProceedingJoinPoint proceedingJoinPoint;
@Around("@annotation(CheckLevel)")
public Object validateAspect(ProceedingJoinPoint pjp) throws Throwable {
this.proceedingJoinPoint = pjp;
MethodSignature signature = (MethodSignature) this.proceedingJoinPoint.getSignature();
Method method = signature.getMethod();
PermissionLevel validateAction = method.getAnnotation(CheckLevel.class);
int level = validateAction.level();
System.out.println("Level is: " + level);
}
}
So now lets see what we have done one by one in here
Now lets see how our custom annotation is being used below:
package com.thecodeprogram.controller;
import com.thecodeprogram.annotation.CheckLevel;
public class UserController {
@CheckLevel(level = 2)
public void deleteData(@PathVariable String id) {
// ......
// Logic implementation
// .....
}
}
As you can see above we just used annotation with a variable. and this annotation will be invoked before method invoked.
That is all
Burak Hamdi TUFAN
Comments