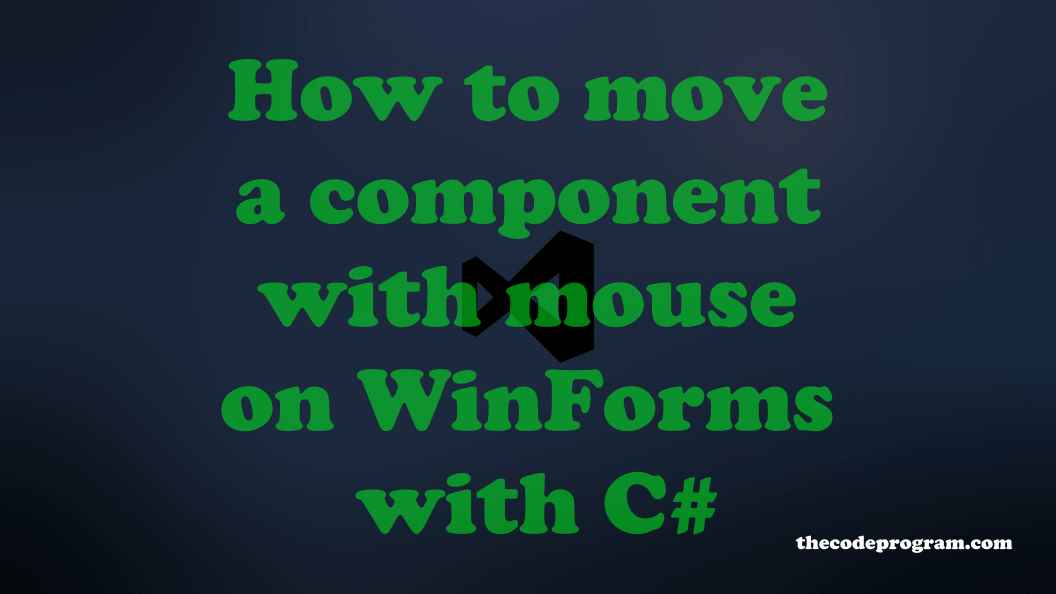
How to move a component with mouse on WinForms with C#
Hello everyone, in this article we are going to see how can we move a component like button, label or a textbox with mouse on the WinForms with C#. We will change its location with mouse moving.Let's get started.
Here is the steps :
Firstly we are going to create a variable that holds the moving State is enabled or not. Also we need to hold the initial Clicked Point to subtract it. Else the cursor will hold the component from its left upper point.
private bool enableMoving = false;
private Point initialClickedPoint;
And then we need to manage above variables when mouse down and mouse up events.
private void btnButton_MouseDown(object sender, MouseEventArgs e)
{
enableMoving = true;
lblState.Text = "Moving Enabled";
initialClickedPoint = e.Location;
}
private void btnButton_MouseUp(object sender, MouseEventArgs e)
{
enableMoving = false;
lblState.Text = "Moving Disabled";
}
When we pressed the button, the moving action will be allowed and also it will update the label as enabled. Also it will load the clicked location to the initialClicked Point variable. We are going to use this during the mouse moving.
Now we are ready to make movement. In here there are to methods:
//Assigning new point to the component location property.
btnButton.Location = new Point(e.X + btnButton.Left - initialClickedPoint.X, e.Y + btnButton.Top - initialClickedPoint.Y);
//Changing the Left and Top values of the component
btnButton.Left = e.X + btnButton.Left - initialClickedPoint.X;
btnButton.Top = e.Y + btnButton.Top - initialClickedPoint.Y;
Both methods are same. During the movement of the mouse first I will check the movement is enabled and if it is enabled I will update the location of the button.
private void btnButton_MouseMove(object sender, MouseEventArgs e)
{
if (enableMoving) // suruklenmedurumu==true ile aynı.
{
btnButton.Location = new Point(e.X + btnButton.Left - initialClickedPoint.X,
e.Y + btnButton.Top - initialClickedPoint.Y);
}
}
Our program is ready.
You can make you movings on the windows forms now.
Burak Hamdi TUFAN
Tags
Share this Post
01/04/2021
How to Create Custom Widget in QT C++
22/02/2022
Operator Overloading in C++
23/04/2022
Comments