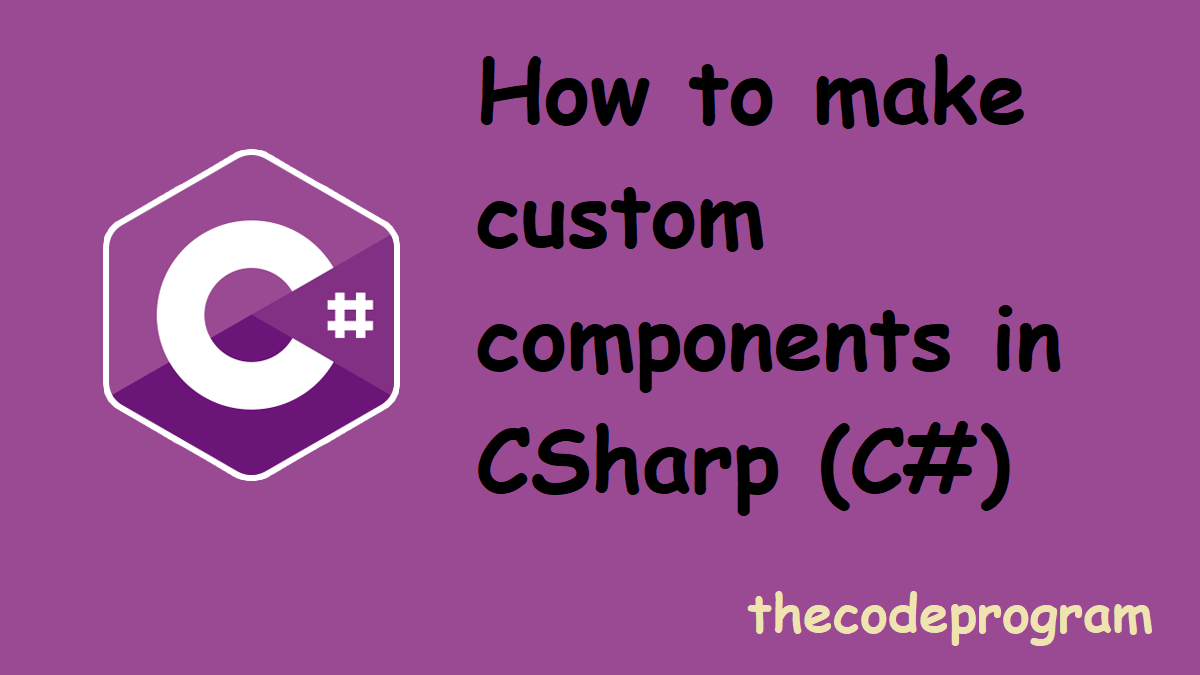
How to create custom components in CSharp (C#)
Hello everyone, in this article we are going to talk about how can we create a custom component in Visual Studio and C#. We are going to make a custom digital clock example.Let's get started.
I always keep my projects tidied up. First create a folder in your project. We are going to create our components classes in this folder. Below image you can find how can we do it.
And then add a Custom Control in this folder. This control class will be our component. Below image you can find it.
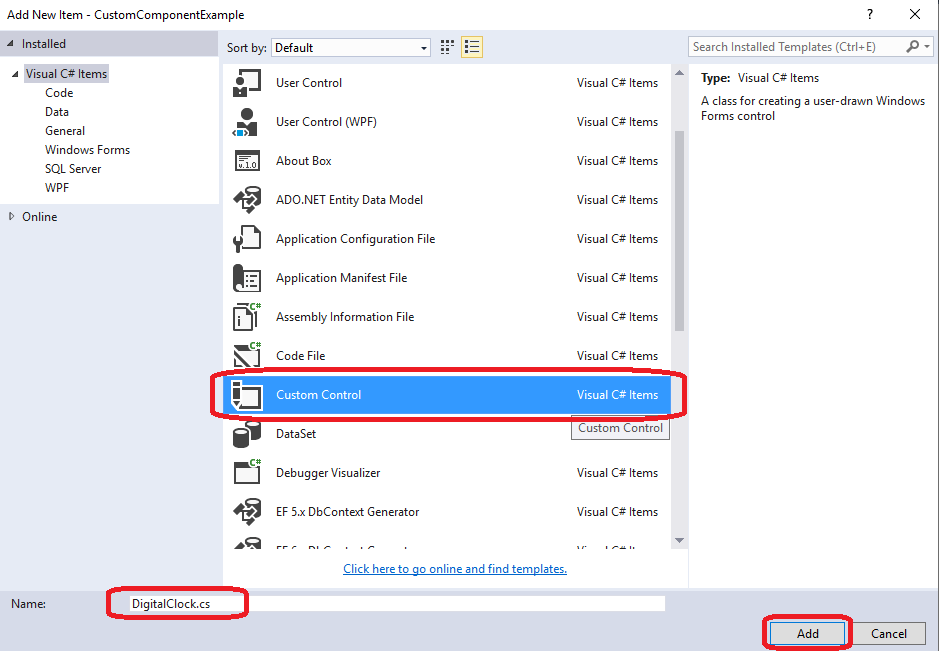
Below code block will be generated automatically:
namespace CustomComponentExample.TheComponent
{
partial class DigitalClock
{
/// <summary>
/// Required designer variable.
/// </summary>
private System.ComponentModel.IContainer components = null;
/// <summary>
/// Clean up any resources being used.
/// </summary>
/// <param name="disposing">true if managed resources should be disposed; otherwise, false.</param>
protected override void Dispose(bool disposing)
{
if (disposing && (components != null))
{
components.Dispose();
}
base.Dispose(disposing);
}
#region Component Designer generated code
/// <summary>
/// Required method for Designer support - do not modify
/// the contents of this method with the code editor.
/// </summary>
private void InitializeComponent()
{
components = new System.ComponentModel.Container();
}
#endregion
}
}
We are going to need some variables in this example. We will make a digital clock component. We will define hour, minute and second variables. We will show them in our component now.
//we will update this variables from upper class.
public int val_hour = 0;
public int val_minute = 0;
public int val_second = 0;
//we do not need this update from upper class so define as private
private string current_clock = "00:00:00";
We are going to show somethingon our form. It means we are going to paint something on form. So we need to add a protected function below to paint our component :
protected override void OnPaintBackground(PaintEventArgs pevent)
{
base.OnPaintBackground(pevent);
}
Now we are ready to show something on screen. Below code block will draw the clock information on screen.
//we will update this variables from upper class.
public int val_hour = 0;
public int val_minute = 0;
public int val_second = 0;
private string str_hour = "00";
private string str_minute = "00";
private string str_second = "00";
//we do not need this update from upper class so define as private
private string current_clock = "00:00:00";
protected override void OnPaintBackground(PaintEventArgs pevent)
{
base.OnPaintBackground(pevent);
//To prevent painting single character...
str_hour = val_hour < 10 ? "0" + val_hour : val_hour.ToString();
str_minute = val_minute < 10 ? "0" + val_minute : val_minute.ToString();
str_second = val_second < 10 ? "0" + val_second : val_second.ToString();
current_clock = str_hour + ":" + str_minute + ":" + str_second;
pevent.Graphics.DrawString(current_clock,
new Font("Comic Sans MS", 32), Brushes.Black, new PointF(0, 0));
}
//This function is required to refresh this component
public void refreshClock()
{
this.Refresh();
}
Now let's write some code to show current time on the screen. So what we will do now.
We are going to declare a Timer and set the interval as 1000ms. It will update the clock every 1 second. Below code block will do this.
Timer tmrClock;
private void Form1_Load(object sender, EventArgs e)
{
tmrClock = new Timer();
tmrClock.Interval = 1000;
tmrClock.Tick += TmrClock_Tick;
tmrClock.Start();
}
private void TmrClock_Tick(object sender, EventArgs e)
{
digitalClock1.val_hour = DateTime.Now.Hour;
digitalClock1.val_minute = DateTime.Now.Minute;
digitalClock1.val_second = DateTime.Now.Second;
digitalClock1.refreshClock();
}
Maybe you will need some functions inside your component. If you need that you have to add component inside your namespace. In this case I will do as below:
using CustomComponentExample.TheComponent;
That is all in this article.
Have a good creating custom components
Burak Hamdi TUFAN
Tags
Share this Post
28/07/2020
How to use QT C++ library in C#
26/03/2021
Java ile Queue Arayüzü Anlatımı
05/04/2021
Comments