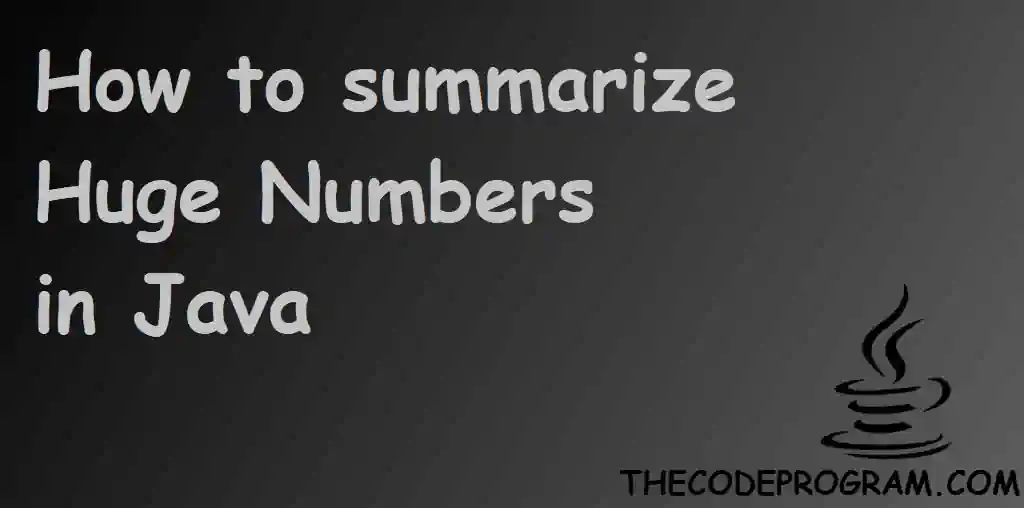
How to summarize Huge Numbers in Java
Hello everyone, in this article we are going to talk about summarising huge numbers which can not be declared with normal decimal numbers and can be declared as string variable types in Java.Let's get started...
So what if we need to make summarising numbers which is greater than double and long maximum values
In here I will use below algorithm, In this algorithm we will consider the numbers as strings and we will make the calculation with all digits like teachers taught us at primary school.
Now let's write some codes.
In here we have three functions. These are a main method to call the function. A summarizeHugeNumbers method to make the calculation with String parameters which hold the huge numbers. And a reverseString to reverse the string in calculation.
public class SummarizeHugeNumbers {
public static void main(String[] args) {
}
public static void summarizeHugeNumbers(String _no1, String _no2){
}
public static String reverseString(String word){
}
}
Firstly we are going to write summarize method.
Below code block will have two String parameters which pass the numbers which be summarized. And then reverse them firstly. This is required to start to summarize from Least Significant Digit. We will re-reverse the number after calculation.
And then we are going to make them same length, so we will add zero's until they are equal. And then we are going to make the calculation digit by digit. If summarisation of digits greater than 10 it will pass the 1 to next value.
public static void summarizeHugeNumbers(String _no1, String _no2){
String output = "";
//reversed number variables
String rno1, rno2;
//if summarized digits more than 10 it will move one next
boolean hasDecimal = false;
//firstly we need to reverse
rno1 = reverseString(_no1);
rno2 = reverseString(_no2);
//find the for loop size according to number character count
int loopSize = rno1.length() >= rno2.length() ? rno1.length() : rno2.length();
//Fill with 0 rest of decimal areas and make them equal lenght
while(rno1.length() != rno2.length() ){
if(rno1.length() < rno2.length()) rno1 += "0";
else if(rno1.length() > rno2.length()) rno2 += "0";
}
for(int i=0; i<loopSize; i++){
//summarize the decimals first
int res = Integer.parseInt(rno1.charAt(i) + "") + Integer.parseInt( rno2.charAt(i)+ "" );
res += hasDecimal ? 1 : 0; //add 1 if hasDecimal
hasDecimal = (res > 9);//set the decimal if bigger than 10
//calculate and add the decimal to output variable
int decimal = res % 10;
output += decimal + "";
}
output = reverseString(output);
System.out.println(output);
}
Now below we will see the reverseString method.
This method first define the output variable and then start to add the chars from end of string.
public static String reverseString(String word){
//First define the output variable
String output = "";
//then start to add char by char to output variable
for(int i=word.length()-1; i>=0; i--){
output += word.charAt(i);
}
return output;
}
public static void main(String[] args) {
String no1 = "12452452452452452452452452425245245245254245245243252234356546765536456785673578568468452452452452";
String no2 = "3454543245454545245452453245453453245454837827245245234524524524545242452452455242452452424524524532453245245245245234532453245345324";
summarizeHugeNumbers(no1, no2);
}
That is all in this article.
You can reach the code on Github via : https://github.com/thecodeprogram/TheSingleFiles/blob/master/SummarizeHugeNumbers.java
Burak Hamdi TUFAN.
Comments