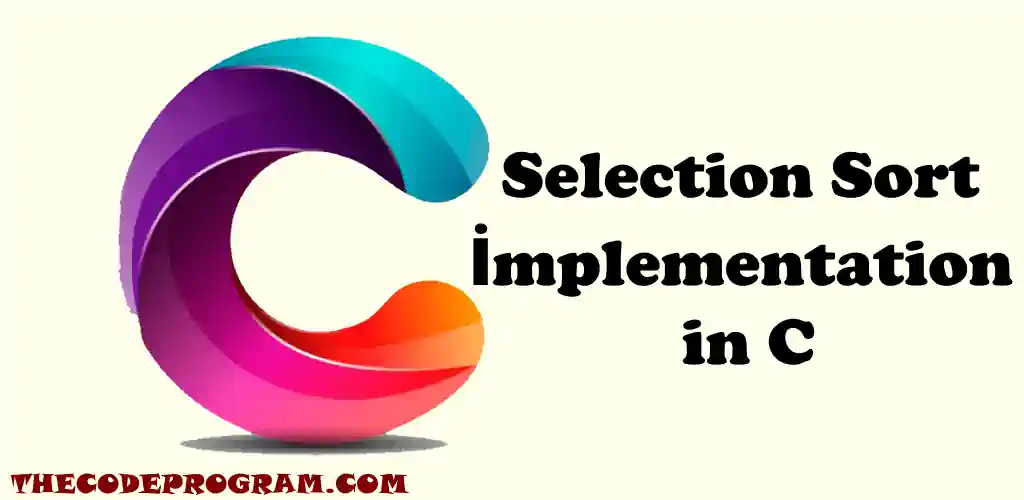
Selection Sort İmplementation in C
Hello everyone, in this article we are going to see implemention of Selection Sort method with C language on an array which filled by random numbers. Sometimes you can not use collections and you may need this method.Let's begin.
Be carefull
All of below methods are in int main() { ... } function.
In here first we generate random numbers and fill the array.
All of below methods are in int main() { ... } function.
We have to generate numbers based on time. Because time is always changing , if we do not do this, program will always generate same numbers. Here we set this and declare the array which holds the generated numbers.
time_t t;
srand((unsigned) time(&t));
int numbers[6];
Here we are going to generate 6 numbers and they will be between 0 - 100. Then we control the generated number if added the array before. If not then go else If generated number is exist in the array then we are setting the flag as bit-0 to prevent adding again. And last if there is no problem we are adding the number at specified index
for(int i = 0 ; i < 6 ; i++ ) {
int adding_ok = 1;
int temp_rand = rand() % 100;
for(int j=0; j < i; j++)
{
if(temp_rand == numbers[j])
{
adding_ok = 0;
i--;
}
}
if(adding_ok == 1)
numbers[i] = temp_rand;
}
Until here our array is ready and we can implement selection sort method,
Below variable will keep the minimum values' index
int min_index;
Here we are going to controll all values in the array. At the begining we have selected the first value so our last kontrol index is 1 lack of array size
In for loop we are going to find the minimum number in the index, So we need to control the indexes after our selected index. Because we assume the little indexes have been ordered from small to bigger. After control it with if we are selecting the index according to being small around rest of numbers.
The important part of implementation is : we are replacing the numbers to prevent deleting them with new order.
for (int i = 0; i < 5; i++)
{
min_index = i;
for (int j = i+1; j < 6; j++)
if (numbers[j] < numbers[min_index])
min_index = j;
int temp = numbers[min_index];
numbers[min_index] = numbers[i];
numbers[i] = temp;
}
And print at console screen
for(int i = 0 ; i < 6 ; i++ ) {
printf("%d\n", numbers[i] );
}
That is all in this article.
You can find the example c file on Github via : https://github.com/thecodeprogram/TheSingleFiles/blob/master/selection_sort_implementation_in_c.c
Now you are good to implemet selection sort method to your projects.
Burak Hamdi TUFAN
Tags
Share this Post
18/04/2020
Comments