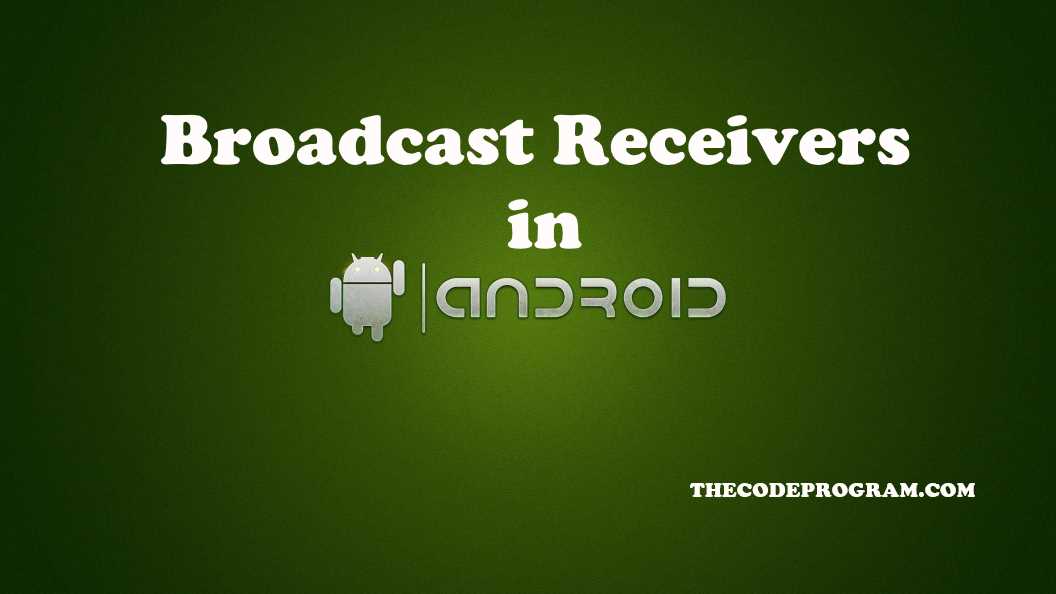
Broadcast Receivers in Android
Hello everyone, in this article we are going to talk about Broadcast Receivers in android and make an example with java. We will make an example about Broadcast Receivers. We will confiure a communication between activity and android services.Now Let's get started.
First I will create a service. You can see how to create and use android service from this link : https://thecodeprogram.com/explanation-of-android-services-with-example. Now we will setup our BroadCast Receivers.
In my MainActivity I will define a BroadCast Receiver and set a filter for my messaging.
Below code block you can see it.
final private BroadcastReceiver mMessageReceiver = new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
String message = intent.getStringExtra("message");
if(message.equals("update_nowplaying"))
{
// run the commands when the message is update_nowplaying
}
else if(message.equals("closeApplication"))
{
finishAffinity();
}
Log.d("Breadcast Receiver", "received message: " + message);
}
};
Also, if we want to pause when we close the or open another application we should stop the messaging. Because we have to take care about device battery. To save energy we should stop the broadcast register. So we should use onStop(), onResume() and onDestroy().
Below code block you can see it:
MainActivity.java
public class MainActivity extends AppCompatActivity {
final private BroadcastReceiver mMessageReceiver = new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
String message = intent.getStringExtra("message");
if(message.equals("update_nowplaying"))
{
// run the commands when the message is update_nowplaying
}
else if(message.equals("closeApplication"))
{
finishAffinity();
}
Log.d("Breadcast Receiver", "received message: " + message);
}
};
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
@Override
protected void onDestroy() {
super.onDestroy();
LocalBroadcastManager.getInstance(getAppContext()).unregisterReceiver(mMessageReceiver);
}
@Override
public void onResume() {
super.onResume();
LocalBroadcastManager.getInstance(getAppContext()).registerReceiver(mMessageReceiver,
new IntentFilter("theBroadcastEvent"));
}
@Override
protected void onPause() {
// Unregister since the activity is not visible
LocalBroadcastManager.getInstance(getAppContext()).unregisterReceiver(mMessageReceiver);
super.onPause();
}
}
Now our activity is ready. We have to configure our service. Below code block you can see the service class.
BackService.java
public class BackService extends Service {
BroadcastReceiver serviceBrodcastReceiver;
@Override
public void onCreate() {
serviceBrodcastReceiver=new BroadcastReceiver() {
@Override
public void onReceive(Context arg0, Intent arg1) {
//Get the value and use it from intent
int value=arg1.getExtras().getInt("key");
//and use it at wherever
}
};
registerReceiver(serviceBrodcastReceiver, new IntentFilter("theBroadcastEvent"));
}
@Override
public void onDestroy() {
super.onDestroy();
//.....
//unregister all broadcast receivers
LocalBroadcastManager.getInstance(getAppContext()).unregisterReceiver(serviceBrodcastReceiver);
//.....
}
}
Now our service and activity are ready, when we trigged a message via Broadcast, our message will go everywhere with this Bradcast.
Our article is end here for now.
Have a good Broadcasting.
Burak hamdi TUFAN
Comments