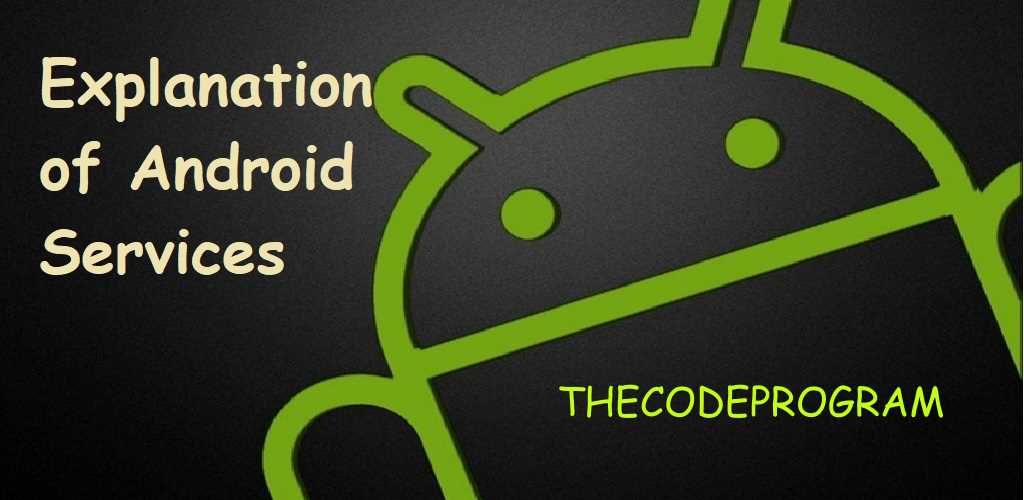
Explanation of Android Services with Example
Hello everyone, in this article we are going to talk about Android Services. How to start, manage, use and stop services from our Android Activity. We will make an example about services.Now, Let's get started.
A small description of Services:
A Service is an application component which performs long-running operations in the background without a user interface. It will continue the operation even if the user switch the another program. Also we can bind a component to this related service to work together. So you can play a music or read a file or check the server whatever you want in the background.
First create a class like below image. I named it as TheService. After creating the class extend this class with Service superclass. And also I recommend you to add constructor block.
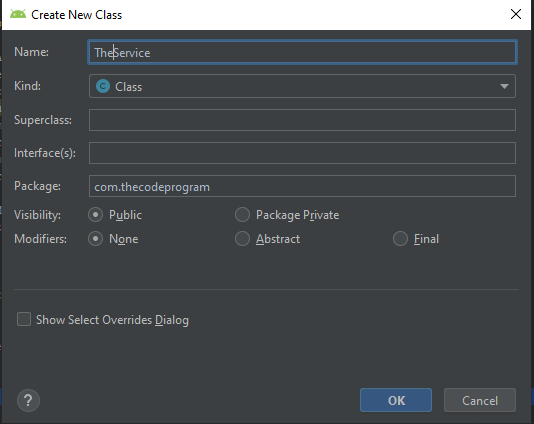
TheService.java
package com.thecodeprogram.themusic;
import android.app.Service;
import android.content.Intent;
import android.os.IBinder;
import androidx.annotation.Nullable;
public class TheService extends Service {
public TheService()
{
}
@Nullable
@Override
public IBinder onBind(Intent ıntent) {
return null;
}
}
<service android:name=".TheService"
android:enabled="true"
android:exported="true"
android:label="@string/app_name">
</service>
Now we are ready to start and use our service.
First we will check is our class is running and if it is not running we are going to initialize the service and if it is running we will not start our service.
Below code block will check the service is running. At for loop our program will check all ID values of processes and if service running return true, else return false.
private boolean isServiceRunning(Class<?> serviceClass) {
ActivityManager manager = (ActivityManager) getSystemService(Context.ACTIVITY_SERVICE);
for (ActivityManager.RunningServiceInfo service : manager.getRunningServices(Integer.MAX_VALUE)) {
if (serviceClass.getName().equals(service.service.getClassName())) {
return true;
}
}
return false;
}
public class MainActivity extends AppCompatActivity {
Intent theServiceIntent;
TheService theService;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
theService = new TheService();
if(!isServiceRunning(TheService.class))
{
theServiceIntent = new Intent(MainActivity.this, TheService.class);
}
ContextCompat.startForegroundService(MainActivity.this, theServiceIntent);
}
private boolean isServiceRunning(Class<?> serviceClass) {
ActivityManager manager = (ActivityManager) getSystemService(Context.ACTIVITY_SERVICE);
for (ActivityManager.RunningServiceInfo service : manager.getRunningServices(Integer.MAX_VALUE)) {
if (serviceClass.getName().equals(service.service.getClassName())) {
return true;
}
}
return false;
}
}
Above code block will check the service is running and will start depends on the situation.
Also you should bind the service. Lets check how to do it.
As a result our activty and service class will be like below. We will destroy our service and unbind our intent when the program destroyed.
MainActivity.java class
public class MainActivity extends AppCompatActivity {
Intent theServiceIntent;
TheService theService;
boolean isBound = false;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
theService = new TheService();
if(!isServiceRunning(TheService.class))
{
theServiceIntent = new Intent(MainActivity.this, TheService.class);
}
ContextCompat.startForegroundService(MainActivity.this, theServiceIntent);
}
private boolean isServiceRunning(Class<?> serviceClass) {
ActivityManager manager = (ActivityManager) getSystemService(Context.ACTIVITY_SERVICE);
for (ActivityManager.RunningServiceInfo service : manager.getRunningServices(Integer.MAX_VALUE)) {
if (serviceClass.getName().equals(service.service.getClassName())) {
return true;
}
}
return false;
}
final private ServiceConnection myConnection = new ServiceConnection()
{
@Override
public void onServiceConnected(ComponentName className,
IBinder service) {
TheService.MyLocalBinder binder = (TheService.MyLocalBinder) service;
TheService= binder.getService();
isBound = true;
}
@Override
public void onServiceDisconnected(ComponentName name) {
isBound = false;
}
};
@Override
protected void onDestroy() {
super.onDestroy();
unbindService(myConnection);
stopService(theServiceIntent);
}
}
TheService.java
package com.thecodeprogram.themusic;
import android.app.Service;
import android.content.Intent;
import android.os.IBinder;
import androidx.annotation.Nullable;
public class TheService extends Service {
private final IBinder myBinder = new MyLocalBinder();
Intent currentIntent = null;
public TheService()
{
}
public class MyLocalBinder extends Binder {
TheService getService() {
return TheService.this;
}
}
@Override
public IBinder onBind(Intent intent) {
currentIntent = intent;
return myBinder;
}
@Override
public void onDestroy() {
super.onDestroy();
stopForeground(true);
stopSelf();
stopService(currentIntent);
}
}
Our service and activity class are ready.
That is all in this article.
Have a good background operations with services.
Burak Hamdi TUFAN
Comments