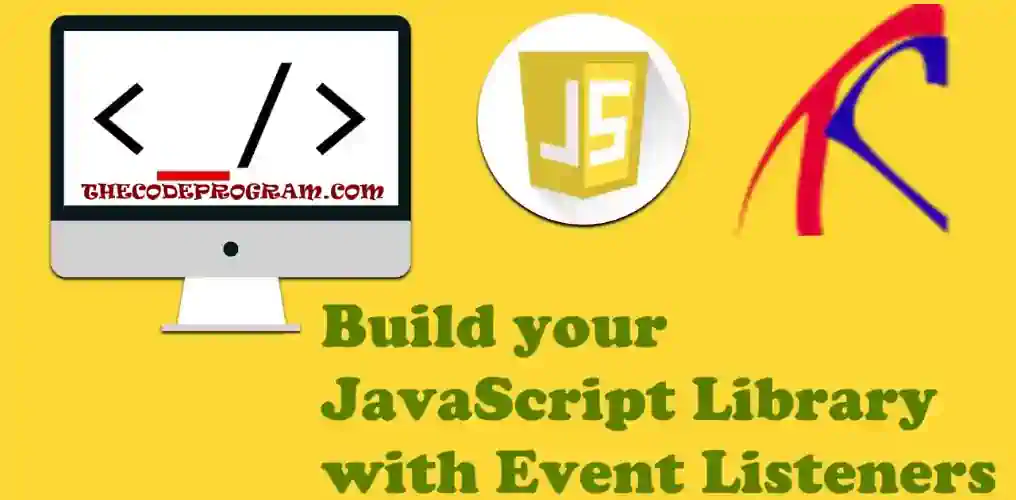
Build your JavaScript Library and Event Listeners
Hello everyone, in this article we are going to talk about creating own JavaScript library. We can use these libraries in every project which supports JavaScript language.Let's get started.
Firstly, Why do we need our own library
During the project development we generally use the exactly same codes even if the project changed. With the library we write the code at one time and after this code block will be usefull and maintainable more. Because we locate our all codes into a library and carry it whereever we want. Also we can manage and reuse our codes easily.And also it will be very cool around the people when you say I have my own library in any programming language :)
Here is the philosophy:
Write Once Use Always
Now let's start to built our own library in Java Script:
First Create a js file for using it as our library file. My Library file name is TheJsLibrary.js .
Here is the one way to create a library:
TheJsLibrary.js
var TheJsLibrary= {
sum: function(a, b) {
return a+b;
},
multiply: function(a, b){
return a*b;
},
subtract: function(a, b){
return a-b;
},
divide: function(a, b){
return a/b;
}
}
Above code block our library file is ready. In web page first we need to import above file like below:
<script type="text/javascript" src="TheJsLibrary.js"></script>
And after importing we are ready to call them in our script area like below:
<script>
console.log("Summarize of 6 and 5 is : " + TheJsLibrary.sum(6,5));
console.log("Multiplyng of 11 and 3 is : " + TheJsLibrary.multiply(11,3));
console.log("Subtraction 5 from 30 is : " + TheJsLibrary.sum(30,5));
console.log("Dividing of 120 by 6 is : " + TheJsLibrary.sum(120,6));
</script>
Now set above method as a click listener on our page. We are going to add this method to a buttons click event listener.
<button id="btnClickMe">Click Me!</button>
<script type="text/javascript" src="TheJsLibrary.js"></script>
<script>
document.getElementById("btnClickMe").addEventListener("click",
function() {
alert("Summarize of 6 and 5 is : " + TheJsLibrary.sum(6,5))
} );
</script>
That is all in this article.
Now you are ready to build your own JavaScript library and use.
Burak Hamdi TUFAN
Tags
Share this Post
17/05/2021
Comments