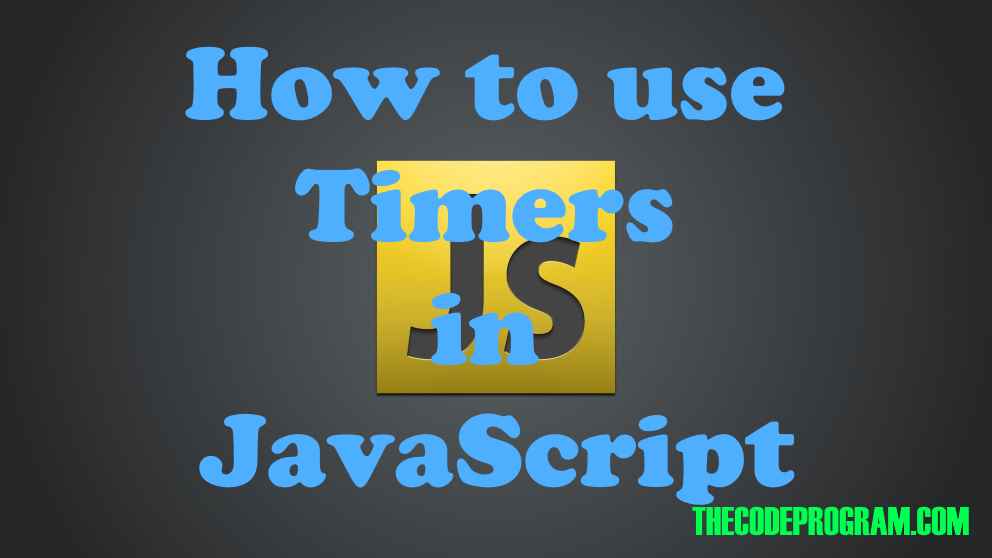
How to use Timer events in JavaScript
Hello everyone, in this article we are going to talk about Timers in JavaScript. We will see a simple description about timers and then we are going to make examples about usages.Lets' begin.
In JavaScript there is window element. This element lets us to access some events and objects in the page. In this article we are going to use window.setTimeout(function, milliseconds) and window.setInterval(function, milliseconds) methods.
setInterval(...) function
window.setInterval(function, milliseconds) has two parameters:
For example below code block will update the date at every seconds:
function fnc_timer() {
var d = new Date();
document.getElementById("div_dateArea").innerHTML = d.toLocaleTimeString();
}
var myInterval = setInterval(fnc_timer, 1000);
We define realted timer as a variable. Because sometimes we may need to stop the intervals. If we do not need to do something after sterting the timer we do not need to define it with variable.
To stop the timer we use clearInterval() method.
Below example you can see how to use it:
var counter = 60;
function fnc_timer() {
counter--;
document.getElementById("div_countDown").innerHTML = counter;
if(counter == 0 ) clearInterval(myInterval );
}
var myInterval = setInterval(fnc_timer, 1000);
Above code block will start to countdown from 60 and when it reach to zero, it will clear the timer interval. This is a countdown example.
setTimeout(...) function
window.setTimeout(function, milliseconds) has two parameters:
Below you can see the example:
function fnc_timeout() {
var d = new Date();
document.getElementById("div_dateArea").innerHTML = d.toLocaleTimeString();
}
var myTimeout = setTimeout(fnc_timeout, 10000);
Above code block will start defined function after ten seconds and start to show the date after 10 seconds.
Also we can stop the timeout with clearTimeout(function) method.
Below code block you will see how to use it.
var counter = 60;
function fnc_timeoutFunction() {
counter--;
document.getElementById("div_countDown").innerHTML = counter;
if(counter == 0 ) clearTimeout(myTimeout );
}
var myTimeout = setTimeout(fnc_timeoutFunction, 1000);
I wish you all healthy days.
Have a good Timing.
Burak Hamdi TUFAN.
Comments