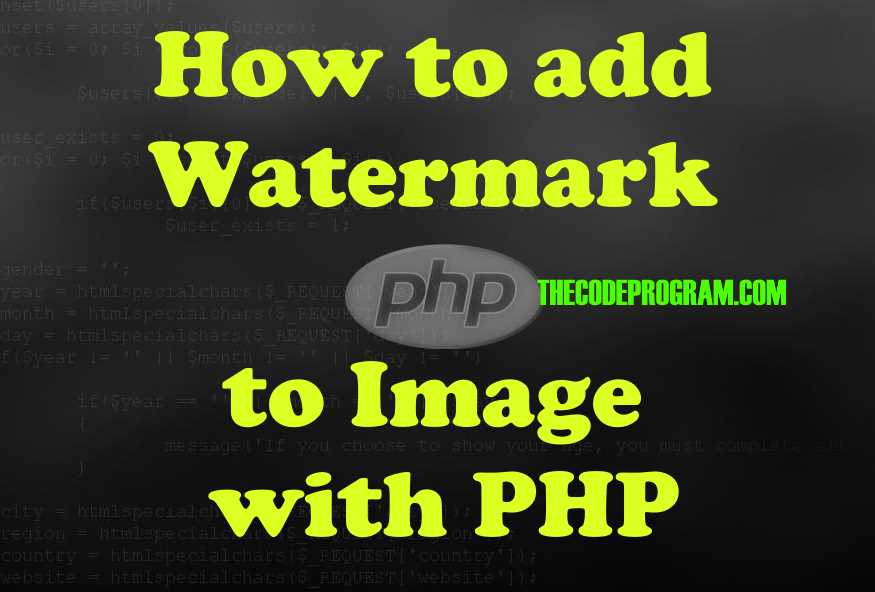
How to add Watermark to Image with PHP
Hello everyone, in this article we are going to see how can we add watermarks to images with PHP. We are going to make a simple description and then we will make an example on this.Let's begin.
I also made this with C# .Net. You can reach via: https://thecodeprogram.com/how-to-add-watermark-to-image-in-c-
In here we are going to make two different ways. We will add a Watermark text as string on an image. Also we are going to add an watermark image on our image.
First we are going to add a watermark image on an image. Then we set the image and watermark image.
First we have to set the output of the page. Here we set it as image. Because we are going to show an image directly. Important thing is we need to create watermark image as png image. Else the transparent ares will be white colored in our watermark image area. And also we need to use a png image to show transparency.
So now we are going to create our image component from the source image and we get its dimensions. Then we specify the position of the watermark. We will locate it right under corner of the image with 20px margin from edges.
Then we are copying the watermark image on created image component with configured locations and dimensions. Lastly show the image on the screen. Do not forget to set header as image of the page. And destroy variables to prevent using memory.
//First we have to set the output of the page.
//Here we set it as image. Because we are going to show an image directly
header('content-type: image/jpeg');
//Then we set the image and watermark image.
$source_image = 'image.jpg';
//Important thing is we need to create watermark image as png image.
//Else the transparent ares will be white colored in our watermark image area.
//And also we need to use a png image to show transparency.
$my_watermark = imagecreatefrompng('watermark.png');
//Then we will get the width and height of the watermark image.
//We will use these dimensions to locate it fit position.
$watermark_width = imagesx($my_watermark);
$watermark_height = imagesy($my_watermark);
//So now we are going to create our image component from the source image.
$image = imagecreatefromjpeg($source_image);
//and we get its dimensions
$image_size = getimagesize($source_image);
//Then we specify the position of the watermark.
//We will locate it right under corner of the image with 20px margin from edges.
$wm_x = $image_size[0] - $watermark_width - 20;
$wm_y = $image_size[1] - $watermark_height - 20;
//Then we are copying the watermark image on created image component with configured locations and dimensions.
imagecopy($image, $my_watermark, $wm_x, $wm_y, 0, 0, $watermark_width, $watermark_height);
//Lastly show the image on the screen. Do not forget to set header as image of the page.
imagejpeg($image);
//And destroy variables to prevent using memory.
imagedestroy($image);
imagedestroy($my_watermark);
Now let's make this process with a text and without a transpaent image.
First get the image And create image resources to process
Then show it on the page
header("Content-type:image/jpeg");
//First get the image
$source_image = "image.jpg";
//And create image resources to process
$image_size = getimagesize($source_image);
$image = imagecreatetruecolor($image_size[0], $image_size[1]);
$image_resource = imagecreatefromjpeg($source_image);
imagecopy($image, $image_resource, 0, 0, 0, 0, $image_size[0], $image_size[1]);
//Extra we need to configure the watermark text
//and font type, font size, color and text angle
$watermarktext = "Thecodeprogram";
$font = "COOPBL.TTF";
$fontsize = "25";
$color = imagecolorallocate($image, 255, 255, 255);
$textangle = 0;
//And location of the text on the image
$wm_x = $image_size[0] - 350 ;
$wm_y = $image_size[1] - 50;
//And then we write the text on the image.
imagettftext($image, $fontsize, $textangle, $wm_x, $wm_y, $color, $font, $watermarktext);
//Then show it on the page
imagejpeg($image);
imagedestroy($image);
That is all in this article.
You can find the examples on Github via:
For image watermarking : https://github.com/thecodeprogram/TheSingleFiles/blob/master/watermark_with_image.php
For text watermarking : https://github.com/thecodeprogram/TheSingleFiles/blob/master/watermark_with_text.php
Have a good watermarking...
I wish you all healthy days.
Burak Hamdi TUFAN
Comments