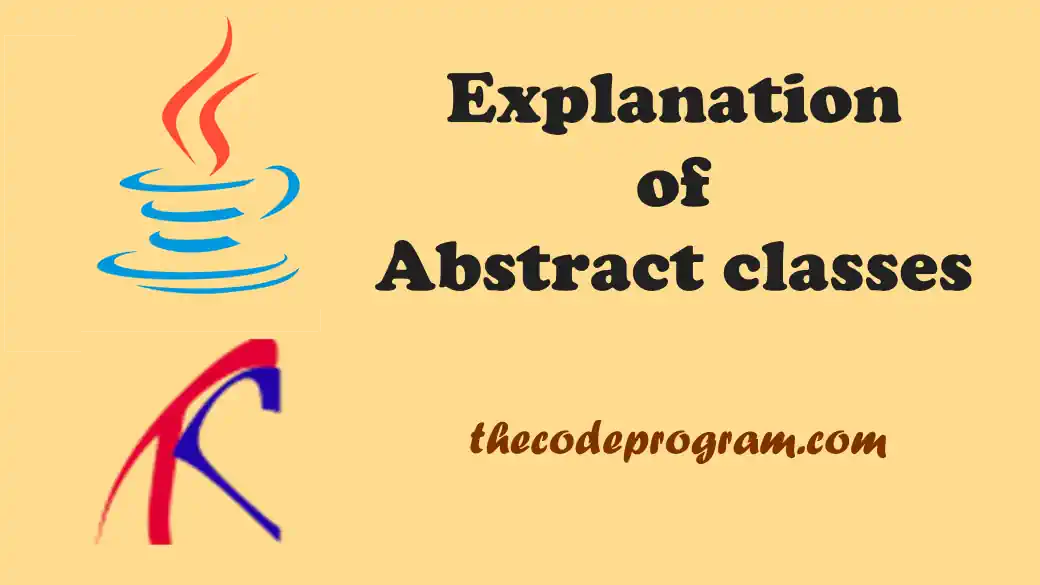
Explanation of Abstract classes in Java
Hello everyone, In this tutorial, we will learn about Java abstract classes and methods with the help of examples. We will also learn about abstraction in Java.Let's begin
What is Abstract class
In Java programming language, an abstract class is a type of class that cannot be instantiated. Abstract classes provide common interface for a group of similar classes because it provides abstract method definitions without body and also provide commonly used methods with implementations for derived classes.
When to use Abstract classes
Abstract classes are helpful with building some hierarchies for classes because they allow you to define a base class with some methods implemented and some methods are abstract to be implemented by the subclasses. This article will show how to create abstract classes and abstract methods in Java programming language.
Abstract Classes
An abstract class in Java is a class that cannot be initialized. Purpose of an abstract class is to serve as a base class for other classes to to be inherited from. Abstract classes can have both abstract and non-abstract methods. The abstract methods in an abstract class do not have an implementation in abstract classes, they must be implemented by derived classes.
For declaring an Abstract class we use abstract keyword before class keyword
Below you will see syntax of Abstract class decleration and usage
abstract class Vehicle {
public void start() {
System.out.println("RRRRRR");
}
public abstract void countTyres();
}
class Car extends Vehicle {
public void countTyres(){
System.out.println("Number of tyre in Car is 4");
}
}
class Bike extends Vehicle {
public void countTyres(){
System.out.println("Number of tyre in Bike is 2");
}
}
class TheExample {
public static void main(String[] args) {
Car c = new Car();
Bike b = new Bike();
c.start();
c.countTyres();
b.start();
b.countTyres();
}
}
Program output will be like below
RRRRRR
Number of tyre in Car is 4
RRRRRR
Number of tyre in Bike is 2
As you can see above Vehicle class is declared as abstract and it has one normal method with implementation and one abstract method without implementation. Two classes inherited Vehicle class and they directly used normal method and they they implemented abstract method according to their requirements.
What is Abstract method?
An abstract method in Java is a method which has no implementation in the abstract class. They only contains the method signature which consists of the method name, return type, and parameters. Abstract methods must be overridden by the subclass.
[access_modifier] abstract [return_type] [method_name](...parameters...)
abstract class Animal {
public abstract void makeSound(double second);
}
As you can see above Animal class is an abstract class and in it, there is abstract method makeSound with parameter. Derived classes will have the implementation of this method
Now let's make an example for better understading.
abstract class Animal {
public abstract void makeSound(double second);
}
class Cat extends Animal {
public void makeSound(double second) {
//...
//... Method body implementation
//...
}
}
As you can see above Cat class extended Animal class and implemented the abstract method body. In thıs way we can specify similar kind of classes and behaviours with different ways.
Conclusion
Abstract classes and abstract methods are very important concepts of Java programming language. Abstract classes provide a way to define a base class that cannot be instantiated, and abstract methods provide a way to declare methods without implementation. They are helpful on building hierarchies of classes and enforcing the implementation of certain methods by the subclass.
Burak Hamdi TUFAN
Comments