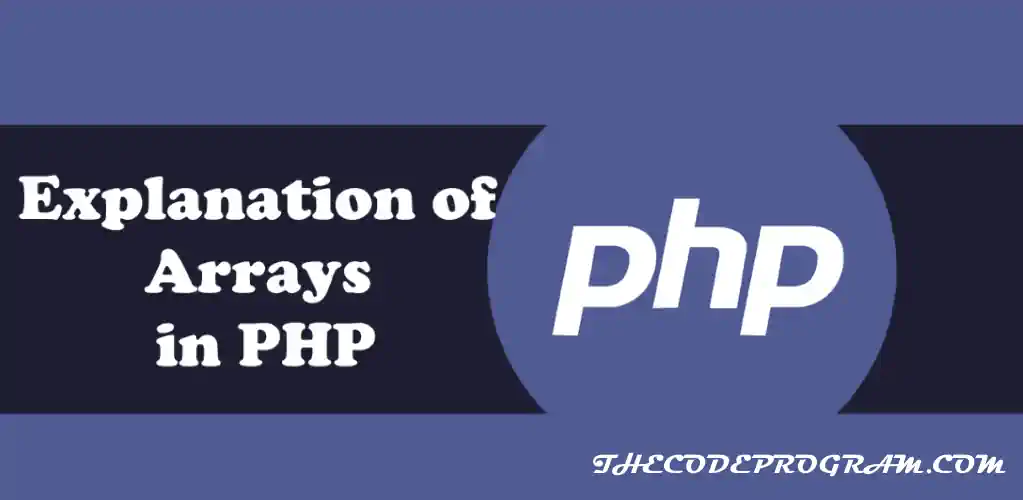
Explanation of Arrays in PHP
Hello everyone, in this article we are going to talk about Arrays in PHP. We will talk about how can we use it and some required array functions which through our examples.Let's begin.
Like so many programming languages we store the datas in arrays in PHP. Array is a variable type which lets to store multiple similar types variables inside ona variable. We keep the datas as list in array.
There are three types of Arrays in PHP:
Let's make examples with these types of arrays :
Indexed Arrays :
At first example we are going to initialize an array with the data which belongs to it. Then we will access these values with their automatically assigned indexes by the array.
// Initialize an indexd arrays with values inside of them
$aircrafts = array("Airbus", "Boeing", "Embraer", "Antonov", "Bombardier", "Cessna");
// Then access the values of above array with their indexes
echo $aircrafts[0] . "\n";
echo $aircrafts[1] . "\n";
echo $aircrafts[2] . "\n";
echo $aircrafts[3] . "\n";
Output will be
Airbus
Boeing
Embraer
Antonov
At second way to initialize an array we can assign the values directly like below. When we assigned a value with indexs inside a variable, it will be array automatically. This is magically side of PHP :). We can access the values like above example with assigned indexes.
// Create an indexed array and assign the values one by one
$engines[0] = "GE90-115";
$engines[1] = "PW4000";
$engines[2] = "TRENT700";
$engines[3] = "V2500";
// then access the values with assigned indexes
echo $engines[0] . "\n";
echo $engines[1] . "\n";
echo $engines[2] . "\n";
echo $engines[3] . "\n";
Output will be
GE90-115
PW4000
TRENT700
V2500
We can also access these arrays elements with loops in PHP. We can different ways to access them. I will show you for, foreach and while loops. Igenerally use for loops but maybe you may in need of other methods. We need count() method to get element count of an array in PHP. We use this method with both for and while loops. We may not be in need to use it with foreach. Because at foreach the operation will be occured for every element in the array.
Remember foreach is an unordered loop. So when you try to use foreach the order may be changed.
//First initialize an array.
$aircrafts = array("Airbus", "Boeing", "Embraer", "Antonov", "Bombardier", "Cessna");
//Accessing them with a for loop
for($i=0; $i < count($aircrafts); $i++){
echo $aircrafts[$i] . "\n";
}
//Accessing them by foreach
foreach ($aircrafts as $ac){
echo $ac . "\n";
}
//To use while loop we need array element count and a current index value
//We need to use count method to get how many items in specified array.
$array_count = count($aircrafts);
$current_index = 0;
while($current_index < $array_count){
echo $aircrafts[$current_index] . "\n";
$current_index++;
}
Output will be same for every loops in this example. That will be below.
Airbus
Boeing
Embraer
Antonov
Bombardier
Cessna
Associative Arrays :
At these type of arrays we assign a specific string type key for all elements in the array. We access these elements with related assigned keys. Below examples we will see the usage of this type of arrays.
At below example we initialize the array with the array method and its key - value pairs. Then we will reach them key values.
//First initialize an array with elements and specified key values
$user_profile = array(
"name"=>"Burak Hamdi",
"surname"=>"TUFAN",
"role"=>"Author",
"web"=>"https://thecodeprogram.com"
);
//Then we access these elements with keys and print them.
echo $user_profile["name"] . "\n";
echo $user_profile["surname"] . "\n";
echo $user_profile["role"] . "\n";
echo $user_profile["web"] . "\n";
Output will be
Burak Hamdi
TUFAN
Author
https://thecodeprogram.com
We can also initialize an array directly assigning a value to a key specified variable. When we do this PHP will recognize it as an array automatically. I told you PHP is a magic :). Below example you will see it.
//First initialize an array with elements and specified key values
$user_profile["name"] ="Burak Hamdi";
$user_profile["surname"] = "TUFAN";
$user_profile["role"] = "Author";
$user_profile["web"] = "https://thecodeprogram.com";
//Then we access these elements with keys and print them.
echo $user_profile["name"] . "\n";
echo $user_profile["surname"] . "\n";
echo $user_profile["role"] . "\n";
echo $user_profile["web"] . "\n";
Output will be
Burak Hamdi
TUFAN
Author
https://thecodeprogram.com
Important :
At this type of arrays do not work with numerical indexes they are working with string type indexes. So we need to get their keys first if we need to use them in a for and while loop. To do this we are going to use array_keys() method and this method will return the keys with its numerical indexes. Then we will get the keys with a numerical index from this key_array. Below example you will see it.
At this type of arrays do not work with numerical indexes they are working with string type indexes. So we need to get their keys first if we need to use them in a for and while loop. To do this we are going to use array_keys() method and this method will return the keys with its numerical indexes. Then we will get the keys with a numerical index from this key_array. Below example you will see it.
Now lets use an associated array with loops. In here we are going to use for and while loops also foreach. We will first initialize an associated array with key-value pairs. To use it foreach we are going to specify them as we specified key-value pairs in the array.
//First initialize an array with key-value pairs.
$user_profile = array(
"name"=>"Burak Hamdi",
"surname"=>"TUFAN",
"role"=>"Author",
"web"=>"https://thecodeprogram.com"
);
// To reach all values in for loop we need an index number but we have string keys.
//So first we need get keys_list of this array then we get their values with these keys
$array_keys = array_keys($user_profile);
$array_count = count($array_keys );
for($i=0; $i < $array_count ; ++$i) {
echo $array_keys [$i] . ' is ' . $user_profile[$array_keys [$i]] . "\n";
}
//Also we can use it with while loop with same logic.
$array_keys = array_keys($user_profile);
$array_count = count($array_keys );
$current_index = 0;
while($current_index < $array_count){
echo $array_keys [$current_index] . ' is ' . $user_profile[$array_keys [$current_index]] . "\n";
$current_index++;
}
// To use it foreach we are going to specify them as we specified key-value pairs
foreach ($user_profile as $element_key => $element_value){
echo $element_key . " is " . $element_value . "\n";
}
The output will be same for every loop. I will share one of them. Output will be:
name is Burak Hamdi
surname is TUFAN
role is Author
web is https://thecodeprogram.com
Multidimensional Arrays :
This type of arrays store another array inside of them. At the above examples we accessed single elements at arrays. In here we are going to access another arrays in an array.
At below example we are going to define an array which has another arrays inside it and then we are going to access these arrays with its assigned numerical indexes.
$aircrafts = array(
array("manufacturer" => "Airbus", "major_model" => "A330", "minor_model" => "300" ),
array("manufacturer" => "Airbus", "major_model" => "A320", "minor_model" => "NEO" ),
array("manufacturer" => "Boeing", "major_model" => "B777", "minor_model" => "300ER" ),
array("manufacturer" => "Beoing", "major_model" => "B737", "minor_model" => "900" ),
);
//We can access the arrays with index at above array:
echo "First AC is " . $aircrafts[0]['manufacturer'] . $aircrafts[0]['major_model'] . $aircrafts[0]['minor_model'] . "\n";
echo "Second AC is " . $aircrafts[1]['manufacturer'] . $aircrafts[1]['major_model'] . $aircrafts[1]['minor_model'] . "\n";
echo "Third AC is " . $aircrafts[2]['manufacturer'] . $aircrafts[2]['major_model'] . $aircrafts[2]['minor_model'] . "\n";
echo "Forth AC is " . $aircrafts[3]['manufacturer'] . $aircrafts[3]['major_model'] . $aircrafts[3]['minor_model'] . "\n";
Output will be:
First AC is AirbusA330300
Second AC is AirbusA320NEO
Third AC is BoeingB777300ER
Forth AC is BoeingB737900
We can also assign these arrays specific keys to reach. At below example we are going to assign string type keys and we are going to access elements with these keys
$aircrafts = array(
"a330" => array("manufacturer" => "Airbus", "major_model" => "A330", "minor_model" => "300" ),
"a320" => array("manufacturer" => "Airbus", "major_model" => "A320", "minor_model" => "NEO" ),
"b777" => array("manufacturer" => "Boeing", "major_model" => "B777", "minor_model" => "300ER" ),
"b737" => array("manufacturer" => "Beoing", "major_model" => "737", "minor_model" => "900" ),
);
//We can access the arrays with index at above array:
echo "First AC is" . $aircrafts["a330"]['manufacturer'] . $aircrafts["a330"]['major_model'] . $aircrafts["a330"]['minor_model'] . "\n";
echo "Second AC is" . $aircrafts["a320"]['manufacturer'] . $aircrafts["a320"]['major_model'] . $aircrafts["a320"]['minor_model'] . "\n";
echo "Third AC is" . $aircrafts["b777"]['manufacturer'] . $aircrafts["b777"]['major_model'] . $aircrafts["b777"]['minor_model'] . "\n";
echo "Forth AC is" . $aircrafts["b737"]['manufacturer'] . $aircrafts["b737"]['major_model'] . $aircrafts["b737"]['minor_model'] . "\n";
Output will be:
First AC is AirbusA330300
Second AC is AirbusA320NEO
Third AC is BoeingB777300ER
Forth AC is BoeingB737900
Now we can access them with loops. In here we are going to use the two loops to access them. First loop is to get all elemenst of the upper array and then we are going to use another loop to get all elements of the inner arrays. If we try to use all types of arrays there will be so many variation. So I will make just one example. The logic is the same.
//First define a multidimensional array.
$aircrafts = array(
array("manufacturer" => "Airbus", "major_model" => "A330", "minor_model" => "300" ),
array("manufacturer" => "Airbus", "major_model" => "A320", "minor_model" => "NEO" ),
array("manufacturer" => "Boeing", "major_model" => "B777", "minor_model" => "300ER" ),
array("manufacturer" => "Beoing", "major_model" => "737", "minor_model" => "900" ),
);
//With for loops we accessed all arrays inside of aircraft array
for($i=0; $i < $aircrafts; ++$i) {
//Assigned every array to a temporary variable
$inner_array = $aircrafts[$i];
// Then we printed every key and value pairs inside these arrays
foreach ($inner_array as $element_key => $element_value){
echo $element_key . " is " . $element_value . "\n";
}
}
Output will be:
First AC is AirbusA330300
Second AC is AirbusA320NEO
Third AC is BoeingB777300ER
Forth AC is BoeingB737900
As you can see we have talked about Arrays in PHP.
That is all in this article.
HAVE A GREATE AIRCRAFT MAINTENANCE ENGINEER DAYS.
Burak Hamdi TUFAN
Tags
Share this Post
27/02/2021
Explanation of Array in JavaScript
23/08/2022
Comments
Hi everybody. Your aticle are so good. I liked. Theres a loop4ever in your code. on the Associative Arrays part., maybe you forgot to use the FUNCTION COUNT($array_keys) on the FOR, at the 2nd Parameter. $array_keys = array_keys($user_profile); //for($i=0; $i < $array_keys; ++$i) { //withount count() function to chek $i < "Strings" in array? for($i=0; $i < count($array_keys); ++$i) { //with count() function to chek $i < to numbers of values in array. echo $array_keys[$i] . ' is ' . $user_profile[$array_keys[$i]] . "\n"; }.
2022/02/07 09:07:29Hello Dear Guest, You are right and I changed the related code block according to your comment. Thank you very much for your dear comment.
2022/02/17 11:41:01