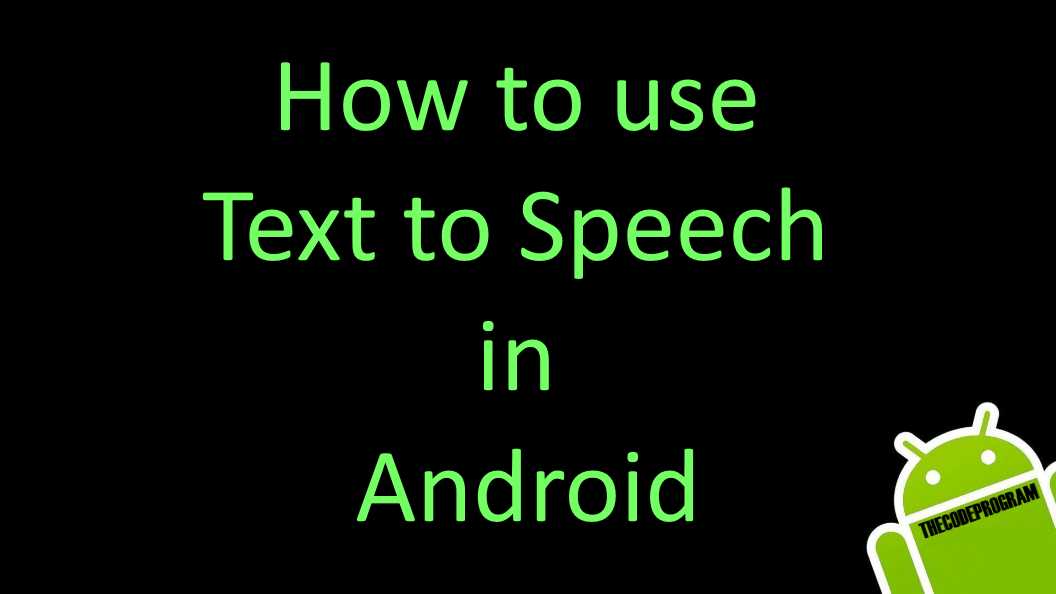
How to use Text to Speech in Android
Hello everyone, in this article we are going to talk about Text to Speech in Android. We will have a discuss on how can we aneble and use this and we will make an example with android studio and java.Let's get started.
First we have to import TextToSpeech library in our activity or fragment. I use Activity on this example. Below code block will import the required library :
import android.speech.tts.TextToSpeech;
And then I will implement the Text to Speech class interfaces to the activity. With this I will use the activity with TextToSpeech operations.
We have declared the TextToSpeech component as tts and we initialized it in onCreate. My activity is ReadActivity and I initialized related component with This class.Below code block you can see it.
public class ReadActivity extends AppCompatActivity implements TextToSpeech.OnInitListener {
private TextToSpeech tts;
String content = "This text will be read by the Android Text to Speech ";
int maxSpeechLength = 0;
Boolean isUnderLimitLenght = false;
Boolean isTtsReady = false;
Boolean isSpeaking = false;
@Override
protected void onCreate(Bundle savedInstanceState) {
tts = new TextToSpeech(ReadActivity.this, ReadActivity.this);
}
}
After Jelly bean android has to check the lenght of the Textinput which will be read and if it is under the limit, it can read it. So first we need to check if the device is after jelly bean and if the text lenght is under the Maximum limit of the TextToSpeech. You should make this control befor the speech, I will do it in onCreate at this example
Below code block will do this:
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN_MR2) {
maxSpeechLength = tts.getMaxSpeechInputLength();
if (content .length() > maxSpeechLength ) {
isUnderLimitLenght = false;
}
else {
isUnderLimitLenght = true;
}
}
After this if my device can read the text, I will check the text is a real text. So, I mean the text has a language and not a random text. After controlling this, we will be ready to make our device read the text.
Below onInit function belongs to the TextToSpeech interface. This function fist check if the TextToSpeech initialized successfully and then it will check the text for language which I specified. I specified here Locale.US. And if there is no problem we will be ready to Make our device to read the text.
@Override
public void onInit(int i) {
if (i == TextToSpeech.SUCCESS) {
int result = tts.setLanguage(Locale.US);
if (result == TextToSpeech.LANG_MISSING_DATA
|| result == TextToSpeech.LANG_NOT_SUPPORTED) {
Log.e("TTS", "This Language is not supported");
isTtsReady = false;
} else {
btnTextToSpeech.setEnabled(true);
isTtsReady = true;
}
} else {
isTtsReady = false;
Toast.makeText(this, "Your device do not support TextToSpeech....", Toast.LENGTH_SHORT).show();
}
}
Now as we are ready we can start tthe speech. Below code block will do this.
First I have checked the my TextToSpeech is speaking, if it is not speaking i will load the text to the TextToSpeech and start to speak.
private void textToSpeech() {
if(tts.isSpeaking() == false){
String text = content_content;
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP) {
tts.speak(text, TextToSpeech.QUEUE_FLUSH, null,null);
} else {
HashMap<String, String> map = new HashMap<>();
map.put(TextToSpeech.Engine.KEY_PARAM_UTTERANCE_ID, "TheCodeProgram");
tts.speak(text, TextToSpeech.QUEUE_FLUSH, map);
}
isSpeaking = true;
}
else if(tts.isSpeaking()){
if(tts.isSpeaking()){
tts.stop();
}
btnTextToSpeech.setImageResource(R.mipmap.speech);
isSpeaking = false;
}
}
And also you must stop the TextToSpeech at onDestroy.
@Override
public void onDestroy() {
if (tts != null) {
tts.stop();
tts.shutdown();
}
super.onDestroy();
}
import android.speech.tts.TextToSpeech;
public class ReadActivity extends AppCompatActivity implements TextToSpeech.OnInitListener {
private TextToSpeech tts;
String content = "This text will be read by the Android Text to Speech.";
int maxSpeechLength = 0;
Boolean isUnderLimitLenght = false;
Boolean isTtsReady = false;
Boolean isSpeaking = false;
@Override
protected void onCreate(Bundle savedInstanceState) {
tts = new TextToSpeech(ReadActivity.this, ReadActivity.this);
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN_MR2) {
maxSpeechLength = tts.getMaxSpeechInputLength();
if (content .length() > maxSpeechLength ) {
isUnderLimitLenght = false;
}
else {
isUnderLimitLenght = true;
}
}
else {
isUnderLimitLenght = true;
}
if(isUnderLimitLenght && isTtsReady ){
textToSpeech()
}
}
@Override
public void onDestroy() {
if (tts != null) {
tts.stop();
tts.shutdown();
}
super.onDestroy();
}
@Override
public void onInit(int i) {
if (i == TextToSpeech.SUCCESS) {
int result = tts.setLanguage(Locale.US);
if (result == TextToSpeech.LANG_MISSING_DATA
|| result == TextToSpeech.LANG_NOT_SUPPORTED) {
Log.e("TTS", "This Language is not supported");
isTtsReady = false;
} else {
btnTextToSpeech.setEnabled(true);
isTtsReady = true;
}
} else {
isTtsReady = false;
Toast.makeText(this, "Your device do not support TextToSpeech....", Toast.LENGTH_SHORT).show();
}
}
private void textToSpeech() {
if(tts.isSpeaking() == false){
String text = content_content;
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP) {
tts.speak(text, TextToSpeech.QUEUE_FLUSH, null,null);
} else {
HashMap<String, String> map = new HashMap<>();
map.put(TextToSpeech.Engine.KEY_PARAM_UTTERANCE_ID, "TheCodeProgram");
tts.speak(text, TextToSpeech.QUEUE_FLUSH, map);
}
isSpeaking = true;
}
else if(tts.isSpeaking()){
if(tts.isSpeaking()){
tts.stop();
}
isSpeaking = false;
}
}
}
You can reach the related Java file via https://github.com/thecodeprogram/TheSingleFiles/blob/master/TextToSpeech_Example.java
That is all in this article.
Have a good Making your device speaking.
I wish you all healthy day.
Burak Hamdi TUFAN
Comments