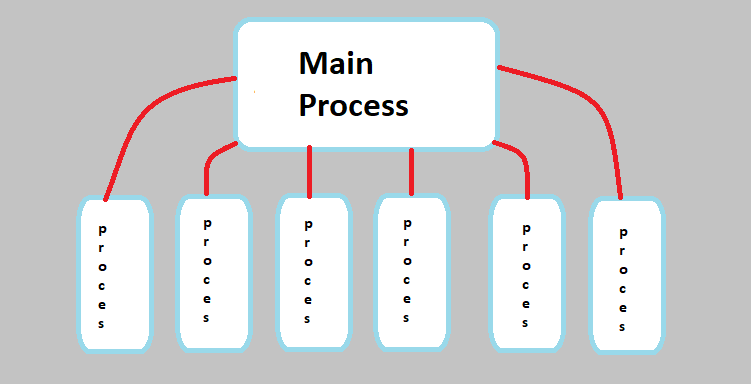
Multi Threading in C# - Working with Multi Channels
Hello everyone, now we are gonna talk about Multi Threading in C#. Firstly I will talk about What Multi Threading is. Then we are going to make examples on multi threading.Multi threading stands for working with multi channel. So there is a mission and our program fulfills this mission with a little processes. With multi process a program can be more powerful and efficient. If a tap fill a pool in an hour, two taps can do this in 30 minutes (if they same types :D ).
In this way, our operations will accelerate twice as three times. Because we will be trading with more than one thread.
. Firstly we need to import Threading library in our project.
using System.Threading;
using System.Threading.Tasks;
After adding the above libraries, we can go to the coding section.
Now we need to specify the methods that will work as thread. It means when thread run specified function will run. We need to prepare this.
public void count1()
{
for (int i = 0; i < 10; i++)
{
Console.WriteLine(i.ToString());
}
}
public void count2()
{
for (int i = 0; i < 10; i++)
{
Console.WriteLine(i.ToString());
}
}
public void count3()
{
for (int i = 0; i < 10; i++)
{
Console.WriteLine(i.ToString());
}
}
We have created three methods and we will call them in three seperate threads. Now take a look at how they work :
Thread tread1= new Thread(count1);
treatd.Start();
Thread tread2= new Thread(count2);
tread2.Start();
Thread tread3= new Thread(count3);
tread3.Start();
The code block above we have created Threads and started them. As parameter of these threads we set methods which we created above section.
As you can see we have declerated and started these methods bounded by these threads.
Result of the program will be similar to below section. This is for my computer, probably result will be different for your computer because of processor working.
1
2
1
2
3
4
1
2
6
7
3
4
5
I did not write here all of them. Now we know how will go on.
What If we want to call same method in seperate threads ?
Of course we can call same method in seperate threads. Let me make an example about this below :
void my_method()
{
for (int i = 0; i < 10; i++)
{
Console.WriteLine(i.ToString());
}
}
Thread tread1 = new Thread(my_method);
tread1.Start();
Thread tread2 = new Thread(my_method);
tread2.Start();
Thread tread3 = new Thread(my_method);
tread3.Start();
As you can see above we have called same function with three seperate threads. If this is a function that must have work more more times, we can do this in shorter time with this threads. Result will be same as above section mixed.
If we want to call same function with different start and end parameter values we can do this like below example :
void function(int start,int end)
{
for (int i = start; i < end; i++)
{
Console.WriteLine(i.ToString());
}
}
Thread tread1 = new Thread(() => function(0,10));
tread1.Start();
Thread tread2 = new Thread(() => function(10,20));
tread2.Start();
We have started this function twice with different parameter values.
1
2
10
11
12
3
14
4
Another method is to start threads with delegates. It means we equalize codes to a delegate and run it in this way. Here is the example of this :
ThreadStart ts = delegate
{
for (int i=0; i<10; i++)
{
for (int i = 0; i < 10; i++)
{
Console.WriteLine(i.ToString());
}
}
};
new Thread(ts).Start();
Our program will find even numbers until a given number. Our program will do this with multi threads.
int count= 0;
int entered_number = Convert.ToInt32(Console.ReadLine());
void check_even_number(int start,int end) //control function
{
for (int i = start; i < end; i++)
{
if (i % 2 == 0)
{
count++;
Console.WriteLine(i.ToString());
}
}
}
int thread_count= entered_number / 1000 + 1;
for (int i = 0; i < thread_count; i++)
{
Thread tread = new Thread(() => check_even_number(thread_count*i,thread_count*(i+1));
tread .Start();
}
We will divide numbers by 1000 to check, entered number will divide by 1000 and create threads to divide this workload.
We have used to for loop to start needed quantity threads.
As you can see above we have created threads as we need quantity. So our mission divided by multiple process, our program gained power and been more efficient.
That's all in this article - Keep in following.
Have a nice coding :)
Burak Hamdi Tufan
Comments