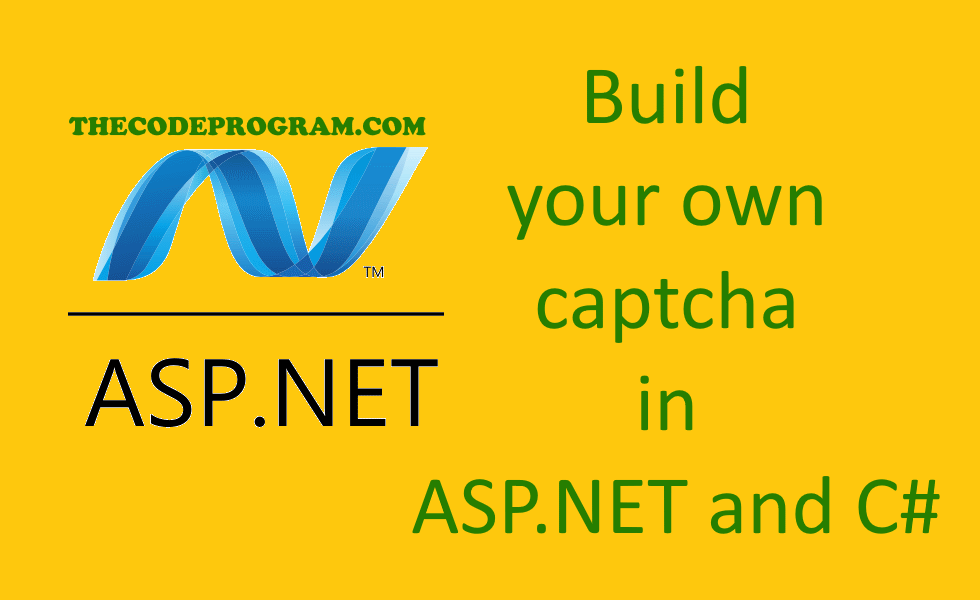
Build your own captcha in ASP.NET and C#
Hello everyone, in this article we are going to talk about building a simple captcha in ASP.NET and C#. We will use Visual Studio to make an example for this article.Let's begin.
First we have to create an ASP.NET project with C# and create a page named Default.aspx. Inside this related page we have to add a Label, a Textbox, an Image and a Button.
We will show our captcha image in Image and we are going to ask the user which enter the captcha inside textbox, after in we will check our caotcha is correct and we will write the result in the Label.
Below code block you can see the design content:
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<asp:Label ID="lblMessage" runat="server" Text=""></asp:Label>
<br />
<asp:TextBox ID="txtCaptchaCode" runat="server"></asp:TextBox>
<br />
<asp:Image ID="imgCaptchaImage" runat="server" />
<br />
<asp:Button ID="btnCheckCaptcha"
runat="server"
onclick="btnCheckCaptcha_Click"
Text="Button" />
</form>
</body>
Now we need to create our image creation code and run them at page startup.
Below code block we will create our captcha code with random characters.
private string generateCaptchaCode(int charCount)
{
Random r = new Random();
string s = "";
for (int i = 0; i < charCount;i++)
{
int a = r.Next(3);
int char;
switch (a)
{
case 1:
char = r.Next(0, 9);
s = s + char.ToString();
break;
case 2:
char = r.Next(65, 90);
s = s + Convert.ToChar(char).ToString();
break;
case 3:
char = r.Next(97, 122);
s = s + Convert.ToChar(char).ToString();
break;
}
}
return s;
}
Now we should create our image creation function. In here we should create variables to set the image width and height.
private string captchaText;
private int width = 400;
private int height = 100;
private Bitmap genetareCaptchaImage()
{
}
Now we will fill the genetareCaptchaImage() code block.
To generate image we will First declare a bitmap and declare graphic from this bitmap and create a rectangle to delegete this image graphic and make operations to draw something. Then create a brush to make some drawings and we need to set the configuration of the brush.
Our rectangle is ready, now we make the text configurations to like font family, font size and color. Then we need to add captcha string to image according to rectangle delegate And the brush that you will write the text. Later We are adding the dots to the image.
When all things are ready we need to dispose the components. This is required for to save resources of the system. Lastly we return created image to our Image component.
Below code block you can see what I mean above:
private Bitmap generateCaptchaImage()
{
//First declare a bitmap and declare graphic from this bitmap
Bitmap bitmap = new Bitmap(width, height, PixelFormat.Format32bppArgb);
Graphics g = Graphics.FromImage(bitmap);
//And create a rectangle to delegete this image graphic
Rectangle rect = new Rectangle(0, 0, width, height);
//And create a brush to make some drawings
HatchBrush hatchBrush = new HatchBrush(HatchStyle.DottedGrid, Color.Aqua, Color.White);
g.FillRectangle(hatchBrush, rect);
//here we make the text configurations
GraphicsPath graphicPath = new GraphicsPath();
//add this string to image with the rectangle delegate
graphicPath.AddString(captchaText, FontFamily.GenericMonospace, (int)FontStyle.Bold, 90, rect, null);
//And the brush that you will write the text
hatchBrush = new HatchBrush(HatchStyle.Percent20, Color.Black, Color.GreenYellow);
g.FillPath(hatchBrush, graphicPath);
//We are adding the dots to the image
for (int i = 0; i < (int)(rect.Width * rect.Height / 50F); i++)
{
int x = rnd.Next(width);
int y = rnd.Next(height);
int w = rnd.Next(10);
int h = rnd.Next(10);
g.FillEllipse(hatchBrush, x, y, w, h);
}
//Remove all of variables from the memory to save resource
hatchBrush.Dispose();
g.Dispose();
//return the image to the related component
return bitmap;
}
We will call these methods at the page load. We will load the created captcha code into session and we will check it when clicked the button.
Below code block you will see the entire code of the program.
Default.aspx.cs
using System;
using System.Collections.Generic;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
public partial class _Default : System.Web.UI.Page
{
private string captchaText;
private int width = 400;
private int height = 100;
private Random rnd = new Random();
protected void Page_Load(object sender, EventArgs e)
{
captchaText = generateCaptchaCode(5);
this.Session["captchaCode"] = captchaText;
imgCaptchaImage.src = generateCaptchaImage();
}
protected void btnCheckCaptcha_Click(object sender, EventArgs e)
{
if (txtCaptchaCode.Text == this.Session["captchaCode"].ToString())
lblmsg.Text = "Captcha is correct";
else
lblmsg.Text = "Captcha is incorrect";
}
private string generateCaptchaCode(int charCount)
{
Random r = new Random();
string s = "";
for (int i = 0; i < charCount; i++)
{
int a = r.Next(3);
int chr;
switch (a)
{
case 0:
chr = r.Next(0, 9);
s = s + chr.ToString();
break;
case 1:
chr = r.Next(65, 90);
s = s + Convert.ToChar(chr).ToString();
break;
case 2:
chr = r.Next(97, 122);
s = s + Convert.ToChar(chr).ToString();
break;
}
}
return s;
}
private Bitmap generateCaptchaImage()
{
//First declare a bitmap and declare graphic from this bitmap
Bitmap bitmap = new Bitmap(width, height, PixelFormat.Format32bppArgb);
Graphics g = Graphics.FromImage(bitmap);
//And create a rectangle to delegete this image graphic
Rectangle rect = new Rectangle(0, 0, width, height);
//And create a brush to make some drawings
HatchBrush hatchBrush = new HatchBrush(HatchStyle.DottedGrid, Color.Aqua, Color.White);
g.FillRectangle(hatchBrush, rect);
//here we make the text configurations
GraphicsPath graphicPath = new GraphicsPath();
//add this string to image with the rectangle delegate
graphicPath.AddString(captchaText, FontFamily.GenericMonospace, (int)FontStyle.Bold, 90, rect, null);
//And the brush that you will write the text
hatchBrush = new HatchBrush(HatchStyle.Percent20, Color.Black, Color.GreenYellow);
g.FillPath(hatchBrush, graphicPath);
//We are adding the dots to the image
for (int i = 0; i < (int)(rect.Width * rect.Height / 50F); i++)
{
int x = rnd.Next(width);
int y = rnd.Next(height);
int w = rnd.Next(10);
int h = rnd.Next(10);
g.FillEllipse(hatchBrush, x, y, w, h);
}
//Remove all of variables from the memory to save resource
hatchBrush.Dispose();
g.Dispose();
//return the image to the related component
return bitmap;
}
}
You can reach the example app via https://github.com/thecodeprogram/AspNet-Captcha-Example
That is all in this article.
Have a great building your own Captcha.
Burak Hamdi TUFAN
Comments