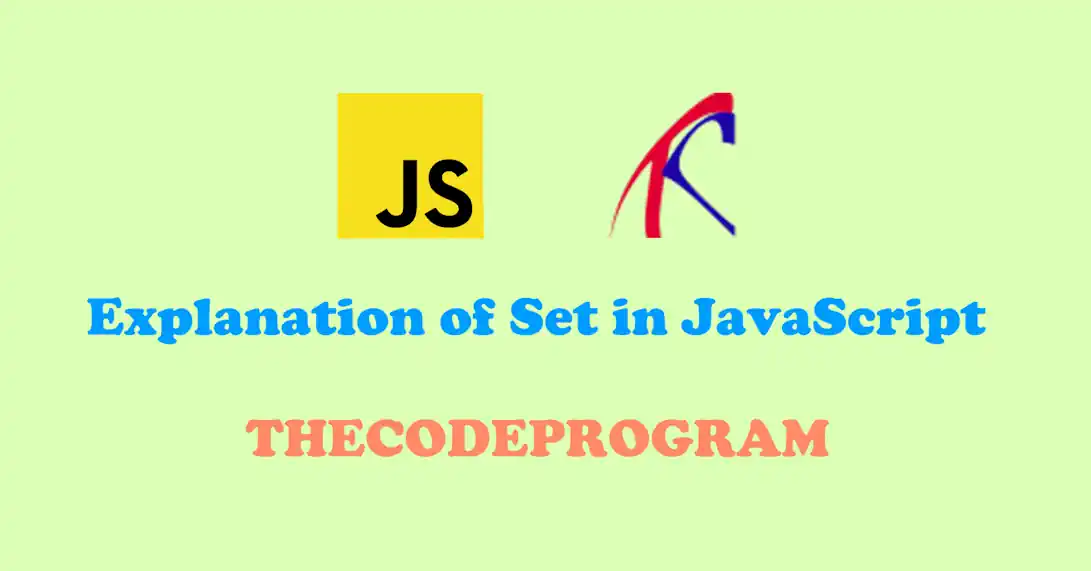
Explanation of Set in JavaScript
Hello everyone, in this article we are going to talk about usage of Set class in JavaScript and we will make some examples for better understanding.Let's get started.
Set is very efficient structure to store datas without duplication. Set is one of very important data structures to store unique information in almost all of programming languages. In JavaScript Set is widely in use to store for storing unique values and it is Introduced with ES6 in JavaScript.
// Initialise Set
const set = new Set();
// Initialise Set from an array
const colors = new Set(['red', 'green', 'blue', 'yellow']);
We can manage the data within Set with add and delete methods of Set class. We can check the number of elements with size property. We can check an element if it is exist in Set with has method.
const set = new Set();
let adding = set.add("red");
console.log(adding) // Set(1) {'red'}
adding = set.add("blue");
console.log(adding) // Set(2) {'red', 'blue'}
let deleting = set.delete("red");
console.log(deleting) // true
deleting = set.delete("green");
console.log(deleting) // false
//red is removed above so it is not exist
console.log(set.has("red")) // false
//blue is still in the set
console.log(set.has("blue")) //true
//One element is removed above. So size is 1
console.log(set.size) // 1
We can iterate the set with for ... of loop. Below you can see the example.
const colors = new Set(['red', 'green', 'blue', 'yellow']);
for (const color of colors) {
console.log(color);
}
We can convert an array to a set and remove the duplicate records from an array with Set.
const colors = ['red', 'green', 'blue', 'green', 'red'];
const set = new Set(colors);
const checkedArray = Array.from(set);
console.log(checkedArray); // ['red', 'green', 'blue']
As a Result
Sets are very efficient way for the collections which needs to store unique values. Sets can be used to remove the duplications from collections and helps us to store unique values. add, delete and has methods are the basic functions of Set to provide these functionalities.
That is all.
Burak Hamdi TUFAN
Comments