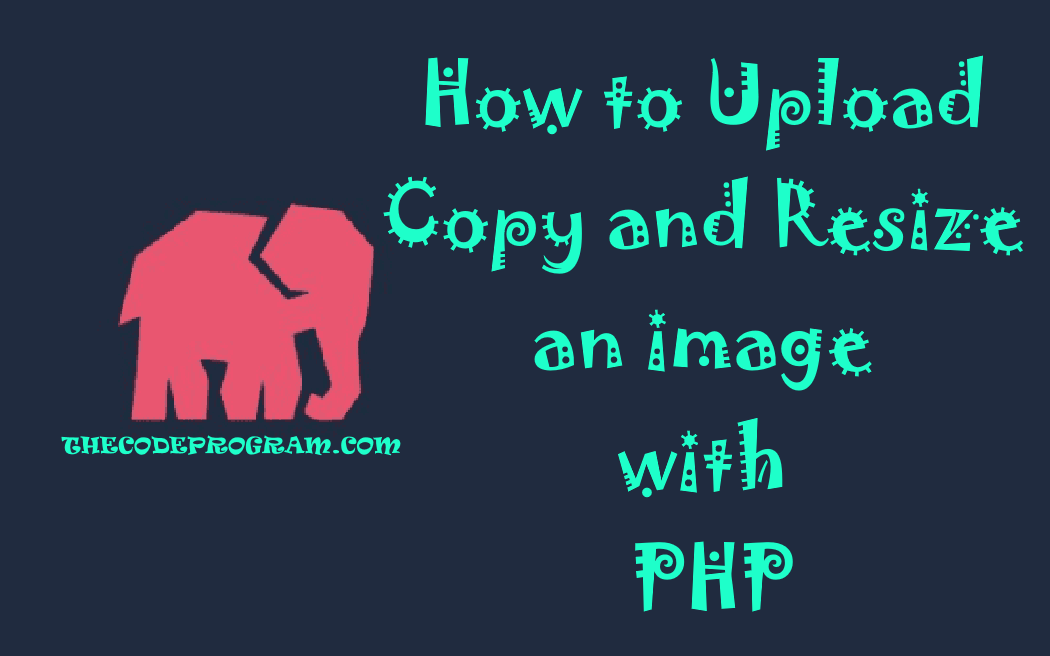
How to Upload Copy and Resize an image with PHP
Hello everyone, in this article we are going to see ow to resize an image in PHP at the server. In this article we are going to upload and image and resize it with new name and locate it in a folder under server.Let's get started.
First we are going to make an upload page and this upload page will send the image to the server. After copying the image it will resize the image with a new name in the server.
Below HTML code block will create a form and inside it a file selector and submit input. This form will send the data to upload_image.php file after submitted.
<form action="upload_image.php" method="post" enctype="multipart/form-data">
Select image to upload:
<input type="file" name="fileToUpload" id="fileToUpload">
<input class="" type="submit" value="Upload Image" name="submit">
</form>
Now after selecting the file, we are going to get the uploaded file and copy it in a folder. Then we are going to resize it with a new name in the server.
At this point we are going to create a method to accomodate our main purpose. This method will copy the image and resize it to target sizes.
First we will check the file is empty or not, if is not empty go on. Then decide the image type is jpeg or pgn orgif or bmp and declare the image to the related image. Get Source image dimensions and Destination image dimensions from parameters of the method. As lastly Prepare and copy image. Set the image quality. I set it as 65% also you can set it from another parameter of the method.
Below function will do what I told above:
function scale_image($image,$target,$target_width,$target_height)
{
//First we will check the file is empty or not, if is not empty go on
if(!empty($image))
{
$source_image = null;
$exploded = explode('.',$image);
$ext = $exploded[count($exploded) - 1];
//Then decide the image type is jpeg or pgn orgif or bmp and declare the image to the related image.
if (preg_match('/jpg|jpeg/i',$ext))
$source_image = imagecreatefromjpeg($image);
else if (preg_match('/png/i',$ext))
$source_image = imagecreatefrompng($image);
else if (preg_match('/gif/i',$ext))
$source_image = imagecreatefromgif($image);
else if (preg_match('/bmp/i',$ext))
$source_image = imagecreatefrombmp($image);
//get Source image dimensions
$source_imagex = imagesx($source_image);
$source_imagey = imagesy($source_image);
//and Destination image dimensions from parameters of the method.
$dest_imagex = $target_width;
$dest_imagey = $target_height;
//Prepare and copy image.
$image2 = imagecreatetruecolor($dest_imagex, $dest_imagey);
imagecopyresampled($image2, $source_image, 0, 0, 0, 0,
$dest_imagex, $dest_imagey, $source_imagex, $source_imagey);
//Set the image quality. I set it as 65% also you can set it from another parameter of the method.
imagejpeg($image2, $target, 65);
}
}
First we have to specify the folder which the image will be uploaded. If the folder is not exist we must create that folder. Below code block you will see how to do it:
$target_dir = "../../img/contents/" . date("Y") . '/' . date("m") . '/' . date("d") . '/';
if (!is_dir($target_dir)) {
mkdir($target_dir, 0777, true);
}
Here we get the uploaded file name and we removed the extension of the file And then we will combine the folder and target file name and again without the file extension. And here we get the file type to decide it is an image or not. To upload image here we should check the format and if it is not an image we cancel the uploading And we declare the target file path with the extention and file name.
//Here we get the uploaded file name and we removed the extension of the file
$file_base_name = substr($_FILES["fileToUpload"]["name"], 0, strrpos($_FILES["fileToUpload"]["name"], "."));
//And then we will combine the folder and target file name and again without the file extension.
$target_file = $target_dir . basename($_FILES["fileToUpload"]["name"]) ;
$original_file_name = substr($target_file, 0, strrpos($target_file, "."));
$uploadOk = 1;
//And here we get the file type to decide it is an image or not.
$imageFileType = strtolower(pathinfo($target_file,PATHINFO_EXTENSION));
//To upload image here we should check the format and if it is not an image we cancel the uploading.
if($imageFileType != "jpg" && $imageFileType != "png" && $imageFileType != "jpeg" && $imageFileType != "gif" ) {
echo "Sorry, only JPG, JPEG, PNG & GIF files are allowed.";
$uploadOk = 0;
}
//And we declare the target file path with the extention and file name.
$target_file = $original_file_name . "." . $imageFileType;
Our configurations are ready now and we are going to upload the file with the built file path.
if (move_uploaded_file($_FILES["fileToUpload"]["tmp_name"], $target_file)) {
echo "Image file was uploaded :". basename( $_FILES["fileToUpload"]["name"]) ;
}
if (move_uploaded_file($_FILES["fileToUpload"]["tmp_name"], $target_file)) {
echo "Image file was uploaded :". basename( $_FILES["fileToUpload"]["name"]). "
";
scale_image($target_file,
substr($target_file,0,strrpos($target_file, ".")) . "_1024x500" . "." . $imageFileType,
1024, 500
);
}
Now we are ready to upload images. Below image you can see the output of the copied images. As you can see I uploaded image and created three copies with different dimensions.

That is all in this article.
Have a good image uploading and resizing.
I wish you all have healthy days.
Burak Hamdi TUFAN
Comments