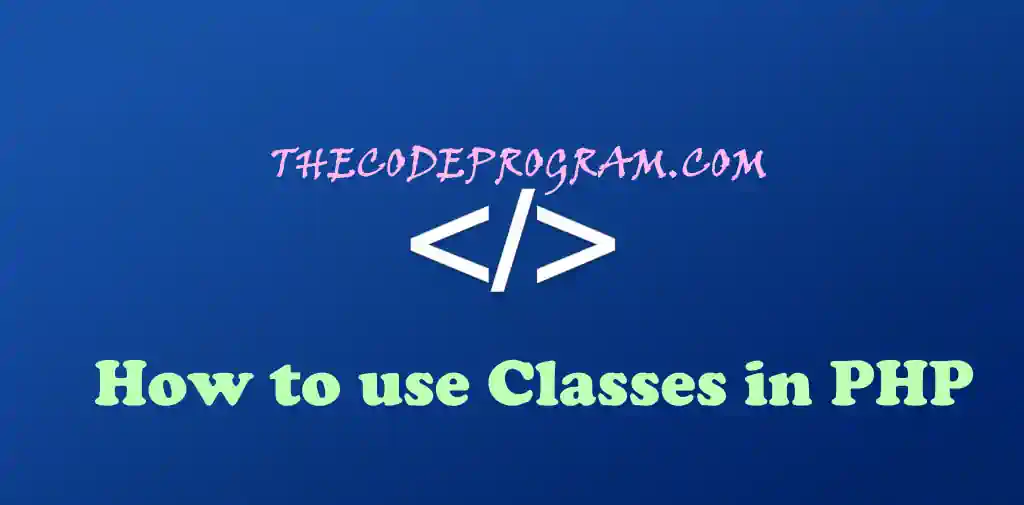
How to use Classes in PHP
Hello everyone, in this article we are going to talk about classes in PHP and how can we use them in our PHP projects with Object Oriented Programming philosophy.Let's get started.
First of All Why should we use classes
For all of Object Oriented Programming lanuages classes are important. Because classes contains the code blocks which reusable and maintainable. Because of this OOP Languages are growing fastly.After PHP5 we are able to use OOP logic and classes in our web projects with PHP. So we can create our classes and use them in any other PHP projects easily and can save time and money :).
In PHP, creating classes are very easy like other programming languages which use classes like Java, C#...
Below code block you can see how to create a PHP class:
class Aircraft{
//Properties of the class
private $brand= "";
private $model = "";
private $year = 0;
//Constructor of the class
public Aircrat($brand, $model, $year){
$this->brand = $brand;
$this->model = $model;
$this->year = $year;
}
//Getters and Setters of the properties of the class
function getBrand(){ return $this->brand; }
function setBrand($brand){ $this->brand = $brand; }
function getModel(){ return $this->model ; }
function setModel($model ){ $this->model = $model ; }
function getYear(){ return $this->year ; }
function setYear($year ){ $this->year = $year ; }
}
As you can see above we have created a class with its properties, constructor, getters and setters within it. Is it really easy isn't it ? :)
Let's use it below...
//First initialize the class with constructor
$ac = new Aircraft("Boeing", "777-200", 1994);
//Print its properties with getter methods
echo "Brand of aircraft : " . $ac->$brand . "\n";
echo "Model of aircraft : " . $ac->$model . "\n";
echo "Year of aircraft : " . $ac->$year . "\n";
Output of above code block will be:
Brand of aircraft : Boeing
Model of aircraft : 777-200
Year of aircraft : 1994
Now use defined above classes' setter methods below:
//Set the properties with through setter methods
$ac->setBrand("Airbus");
$ac->setModel("A320-213");
$ac->setYear("1987");
//Print its properties with getter methods again
echo "Brand of aircraft : " . $ac->$brand . "\n";
echo "Model of aircraft : " . $ac->$model . "\n";
echo "Year of aircraft : " . $ac->$year . "\n";
And below you will see the out put of above code block:
Brand of aircraft : Airbus
Model of aircraft : A320-213
Year of aircraft : 1987
That is all in this article.
Now you care good to use classes in your PHP project.
Burak Hamdi TUFAN
Tags
Share this Post
01/09/2023
Explanation of Set in JavaScript
02/09/2023
Comments